The use of images in documents is a routine operation while working with Microsoft Word. Images give clarity to the content of the document as well as play a vital role in relaying information that is more difficult to put into words. Adding images makes the content easier to understand. As a .NET developer, adding the functionality of inserting images into a Word document is an interesting feature that can add value to your application.
In this article, we will show how to add an image to a Word document from within your .NET application. We’ll be using NPOI API for .NET in C# for this purpose.
Insert Image in Word Document using Microsoft Word
Inserting an image in a Word document is pretty easy. Microsoft Word allows you to insert images in your document and manage its overall layout. You can insert images between text, make these as text background, flow images via text, and many more.
Steps to Insert Image in Document using Microsoft Word
You can use the following steps to insert an image in a Word document using Microsoft Word.
- Open Microsoft Document and select Blank Document. This will open a blank document for you to write something.
- Go to Insert menu > Pictures > This Device for a picture on your PC.
- Select the picture you want to insert in your document. This will insert the image in your document at the cursor location.
- Once you have inserted the picture, you can resize it or move it. You can also wrap text around a picture by selecting. it and then select a wrapping option.
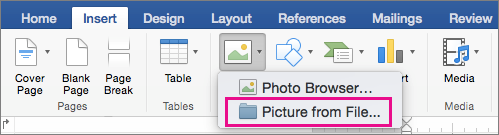
How to Insert Image in Word Document using C#?
Now that we have got the idea of how to insert images using Microsoft Word, we can jump into writing a C# console application to do the same using NPOI API for .NET. Just in case you don’t have much idea about NPOI, you can go through our comprehensive guide for NPOI and its installation guidelines.
Steps to Insert Image in Word Document using NPOI in C#
You can use the following steps to insert an image in a Word document using NPOI API in C#.
- Create an instance of XWPFDocument class
- Create an instance of XWPFParagraph class
- Create an instance of XWPFRun class
- Load and Add the image to XWPFRun instance
- Save the file to disc as DOCX using the XWPFDocument instance
You can use the following C# code sample for the above steps.
//Create document
XWPFDocument document = new XWPFDocument();
XWPFParagraph paragraphy = document.CreateParagraph();
XWPFRun run = paragraphy.CreateRun();
//Insert image and set its size
using (FileStream picFile = new FileStream("dog-puppy.jpeg", FileMode.Open, FileAccess.Read))
{
run.AddPicture(picFile, (int)PictureType.PNG, "image", 300 * 10857, 168 * 12857);
}
//Save the file
using (FileStream file = File.Create("ImageInDocument.docx"))
{
document.Write(file);
}
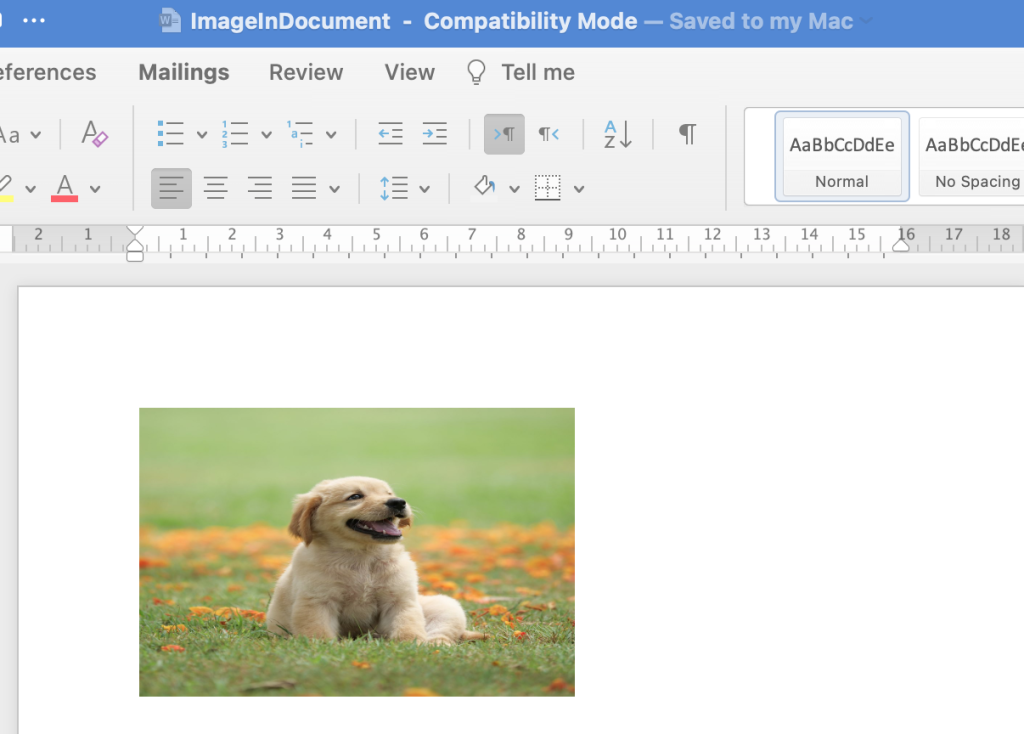
Conclusion
In this article, we had a look at how you can insert an image in a Word document using C#. We used the open-source free API NPOI for .NET for this purpose which is super easy to work with. This series of example articles are targeting to work with NPOI API for Document Processing using NPOI in C#. For more examples, stay tuned.