Install this open-source C# library to insert text into Word documents programmatically. FileFormat.Words is an OpenXML-based API for word document automation.
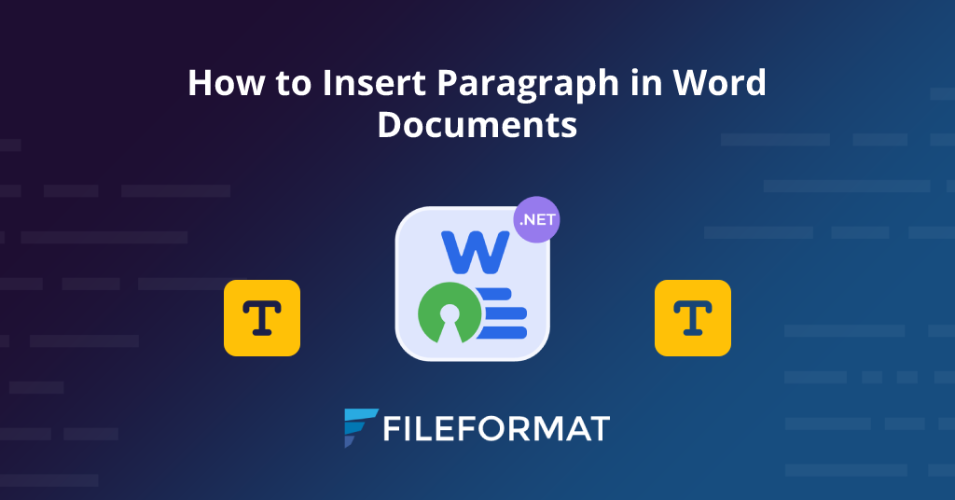
Overview
MS Word documentation automation is no longer a difficult task after the advent of this open-source C# library. FileFormat.Words is a robust .NET library powered by OpenXML. This open-source .NET API is a free library built to create and manipulate Word documents programmatically. Moreover, it not only lets users add paragraphs but also lets users add other elements such as Tables, Images, and more. However, in this blog post, we will learn how to insert paragraph in Word documents using FileFormat.Words. In addition, we will also write a code snippet to see the actual implementation.
We will go through the following points in this article:
Open-Source C# Library Installation
The installation of this Word document automation library is just a command away. So, install this open-source API before you are going to insert text into Word documents programmatically. Therefore, you may set up FileFormat.Words by downloading the NuGet Package or running the following command in the NuGet Package manager.
Install-Package FileFormat.Words
How to Insert Paragraph in Word Documents Programmatically
Now, we are all set to start writing code snippets to insert Paragraph in Word documents using this open-source C# library.
You may follow the steps and the code snippet mentioned below:
- Instantiate an instance of the Document class.
- Initialize the constructor of the Body class with the object of the Document class.
- Instantiate an instance of the Paragraph class.
- Invoke the Text property to set the text of the paragraph.
- Call the AppendChild(paragraph) method to add the paragraph to the MS Word document.
- Invoke the Save method to save the MS Word document onto the disk.
Conclusion
using FileFormat.Words;
namespace Example
{
class Program
{
static void Main(string[] args)
{
// Instantiate an instance of the Document class.
using (Document doc = new Document())
{
//Initialize the constructor of the Body class with the object of the Document class.
Body body = new Body(doc);
// Instantiate an instance of the Paragraph class.
Paragraph para1 = new Paragraph();
// Invoke the Text property to set the text of the paragraph.
para1.Text = "This is a paragraph. This is a paragraph. This is a paragraph. This is a paragraph. This is a paragraph. This is a paragraph. This is a paragraph. This is a paragraph. This is a paragraph. This is a paragraph. This is a paragraph.";
para1.Indent = "300";
para1.FirstLineIndent = "330";
para1.Align = "Left";
para1.LinesSpacing = "552";
// Call the AppendChild(paragraph) method to add the paragraph to the MS Word document.
body.AppendChild(para1);
// Invoke the Save method to save the MS Word document onto the disk.
doc.Save("/Docs.docx");
}
}
}
}
Copy and paste the following code and run the project. Hence, you will see the output as shown in the image below:
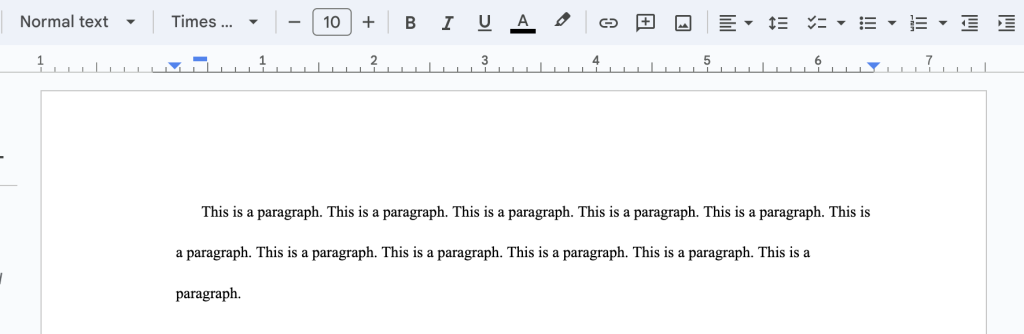
Conclusion
That’s it. We hope you have learned how to insert Paragraph in Word documents using FileFormat.Words. Further, you may customize the font, size, and line spacing of the text of the paragraph. Moreover, you may explore the other cool features of this open-source C# library in the documentation.
Finally, fileformat.com continues to write blog posts on other topics. Moreover, you can follow us on our social media platforms, including Facebook, LinkedIn, and Twitter.
Contribute
Since FileFormat.Words for .NET is an open-source project and is available on GitHub. So, the contribution from the community is much appreciated.
Ask a Question
You can let us know about your questions or queries on our forum.
Frequently Asked Questions – FAQs
How do you insert a paragraph in Word?
You can do that easily using FileFormat.Words. Please follow this link to learn the steps and the code snippet.