Follow this blog post to learn how to add table headers in Word documents programmatically. FileFormat.Words offers rich table creation & manipulation methods.
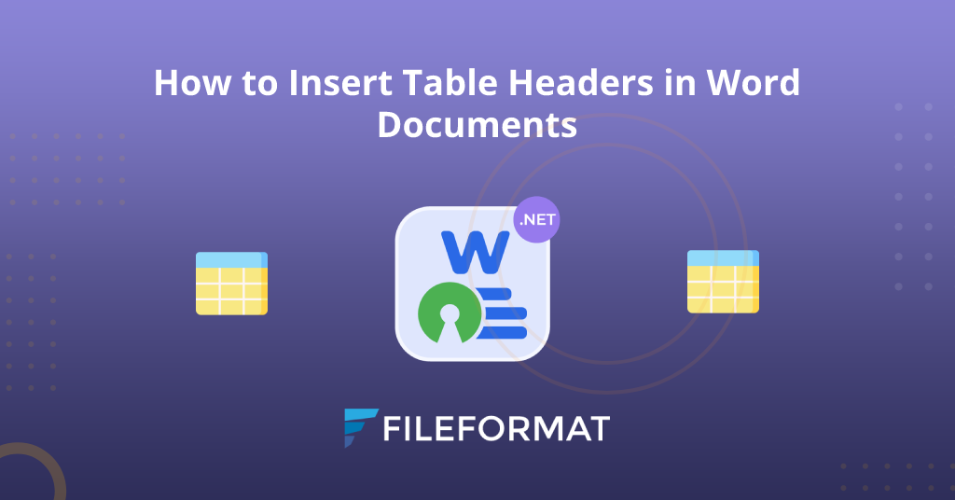
Overview
Data tables are critical elements in MS Word documents. Working with tables is a routine task but what if there are a number of documents with multiple data tables involved? Of course, some sort of automation will badly be needed to automate the repetitive tasks to save time and boost productivity. Therefore, FileFormat.Words is an open-source .NET library to automate Word creation, modification, and processing. In this article, we will explore how to insert table headers in Word documents using this C# API. However, you can visit our previous articles on various topics related to tables in MS Word.
We will go through the following sections in this blog post:
Working With Table Headers – API Installation
The installation procedure of FileFormat.Words for .NET library is a matter of seconds. This enterprise-level .NET API provides a vast stack of features that users can leverage. So, you can download the NuGet Package or run the following command in the NuGet Package Manager.
Install-Package FileFormat.Words
Adding Table Headers in Word Files Programmatically
The installation is completed, the next step is to write the code snippet right away. Further, we can not only create table in Word document but also we can customize the layout of tables programmatically.
You may follow the steps and the code snippet mentioned below:
- Instantiate an object of the Document class.
- Initialize the constructor of the Body class with the Document class object.
- Create an instance of the Table class.
- Set the header of the first column by invoking the TableHeaders method.
- Invoke the Append method to add the rows to the table.
- Call the AppendChild method to add the table to the body of the document.
- The Save method will save the Word document onto the disk.
using FileFormat.Words;
using FileFormat.Words.Table;
namespace Example
{
class Program
{
static void Main(string[] args)
{
// Instantiate an object of the Document class.
using (Document doc = new Document())
{
// Initialize the constructor of the Body class with the Document class object.
Body body = new Body(doc);
// Create an instance of the Table class.
Table table = new Table();
// Initialize the constructor of the TopBorder class to set the border of the top side of the table.
TopBorder topBorder = new TopBorder();
// Invoke the dashed_border method to set the border style and border line width.
topBorder.dashed_border(20);
// To set the border of the bottom side of the table.
BottomBorder bottomBorder = new BottomBorder();
bottomBorder.dashed_border(20);
// To set the border of the right side of the table.
RightBorder rightBorder = new RightBorder();
rightBorder.dashed_border(20);
// To set the border of the left side of the table.
LeftBorder leftBorder = new LeftBorder();
leftBorder.dashed_border(20);
// To set the inside vertical border of the table.
InsideVerticalBorder insideVerticalBorder = new InsideVerticalBorder();
insideVerticalBorder.dashed_border(20);
// To set the inside vehorizontalrtical border of the table.
InsideHorizontalBorder insideHorizontalBorder = new InsideHorizontalBorder();
insideHorizontalBorder.dashed_border(20);
// Create an instance of the TableBorders class.
TableBorders tableBorders = new TableBorders();
// Append the object of the TopBorder class to the object of the TableBorders class.
tableBorders.AppendTopBorder(topBorder);
// Append the object of the BottomBorder class.
tableBorders.AppendBottomBorder(bottomBorder);
// Append the object of the RightBorder class.
tableBorders.AppendRightBorder(rightBorder);
// Append the object of the LeftBorder class.
tableBorders.AppendLeftBorder(leftBorder);
// Append the object of the InsideVerticalBorder class.
tableBorders.AppendInsideVerticalBorder(insideVerticalBorder);
// Append the object of the InsideHorizontalBorder class.
tableBorders.AppendInsideHorizontalBorder(insideHorizontalBorder);
// Initialize an instance of the TableProperties class.
TableProperties tblProp = new TableProperties();
// Invoke the Append method to attach the object of the TableBorders class.
tblProp.Append(tableBorders);
// Create an instance of the TableJustification class
TableJustification tableJustification = new TableJustification();
// Call the AlignLeft method to position the table on left side of the document.
tableJustification.AlignLeft();
// Invoke the Append method to attach the tableJustification object to the tblProp object.
tblProp.Append(tableJustification);
// The AppendChild method will attach the table propertiese to the table.
table.AppendChild(tblProp);
// Create an object of the TableRow class to create a table row.
TableRow tableRow = new TableRow();
TableRow tableRow2 = new TableRow();
// Initialize an istance of the TableCell class.
TableCell tableCell = new TableCell();
Paragraph para = new Paragraph();
Run run = new Run();
// Set the header of the first column by invoking the TableHeaders method.
table.TableHeaders("Country");
run.Text = "England";
para.AppendChild(run);
// Call the Append method to add text inside the table cell.
tableCell.Append(para);
// Create an object of the TableCellProperties table properties
TableCellProperties tblCellProps = new TableCellProperties();
// Set the width of table cell by initializing the object of the TableCellWidth class and append to tblCellProps object.
tblCellProps.Append(new TableCellWidth("2400"));
// Append method will attach the tblCellProps object with the object of the TableCell class.
tableCell.Append(tblCellProps);
TableCell tableCell2 = new TableCell();
Paragraph para2 = new Paragraph();
Run run2 = new Run();
// Invoke the TableHeaders method to set the header of the second column
table.TableHeaders("Capital");
run2.Text = "London";
para2.AppendChild(run2);
tableCell2.Append(para2);
TableCellProperties tblCellProps2 = new TableCellProperties();
tblCellProps2.Append(new TableCellWidth("1400"));
tableCell2.Append(tblCellProps2);
TableCell tableCell3 = new TableCell();
Paragraph para3 = new Paragraph();
Run run3 = new Run();
table.TableHeaders("Population");
run3.Text = "1000000";
para3.AppendChild(run3);
tableCell3.Append(para3);
TableCellProperties tblCellProps3 = new TableCellProperties();
tblCellProps3.Append(new TableCellWidth("1400"));
tableCell3.Append(tblCellProps3);
// Call the Append method to add cells into table row.
tableRow.Append(tableCell);
tableRow.Append(tableCell2);
tableRow.Append(tableCell3);
// create table cell
TableCell _tableCell = new TableCell();
Paragraph _para = new Paragraph();
Run _run = new Run();
_run.Text = "Pakistan";
_para.AppendChild(_run);
_tableCell.Append(_para);
TableCellProperties tblCellProps1_ = new TableCellProperties();
tblCellProps1_.Append(new TableCellWidth("2400"));
_tableCell.Append(tblCellProps1_);
TableCell _tableCell2 = new TableCell();
Paragraph _para2 = new Paragraph();
Run _run2 = new Run();
_run2.Text = "Islamabad";
_para2.AppendChild(_run2);
_tableCell2.Append(_para2);
TableCellProperties tblCellProps2_ = new TableCellProperties();
tblCellProps2_.Append(new TableCellWidth("1400"));
_tableCell2.Append(tblCellProps2_);
TableCell _tableCell3 = new TableCell();
Paragraph _para3 = new Paragraph();
Run _run3 = new Run();
_run3.Text = "2000000";
_para3.AppendChild(_run3);
_tableCell3.Append(_para3);
TableCellProperties tblCellProps3_ = new TableCellProperties();
tblCellProps3_.Append(new TableCellWidth("1400"));
_tableCell3.Append(tblCellProps3_);
tableRow2.Append(_tableCell);
tableRow2.Append(_tableCell2);
tableRow2.Append(_tableCell3);
// Invoke the Append method to add the rows into table.
table.Append(tableRow);
table.Append(tableRow2);
// Call the AppendChild method to add the table to the body of the document.
body.AppendChild(table);
// The Save method will save the Word document onto the disk.
doc.Save("/Users/Mustafa/Desktop/Docs.docx");
}
}
}
}
Copy and paste the above code into your main file and run the program. You will see the output shown in the image below:
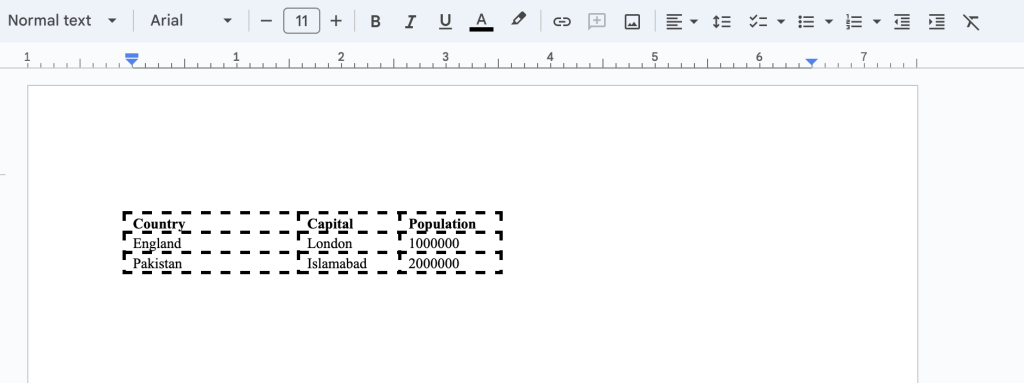
Conclusion
We are ending this blog post here with the hope that you have learned how to insert table headers in Word documents using FileFormat.Words library. In addition, you have gone through the installation process and the code snippet too. Further, there are other practical methods that you can explore in the documentation.
Finally, fileformat.com continues to write blog posts on other topics. Moreover, you can follow us on our social media platforms, including Facebook, LinkedIn, and Twitter.
Contribute
Since FileFormat.Words for .NET is an open-source project and is available on GitHub. So, the contribution from the community is much appreciated.
Ask a Question
You can let us know about your questions or queries on our forum.
Frequently Asked Questions – FAQs
How do you insert a table with headers?
Please follow this link to learn how to insert table headers in C#.