Replace text in Word documents using FileFormat.Words. Use this free & open-source .NET API to perform search and replace text programmatically.
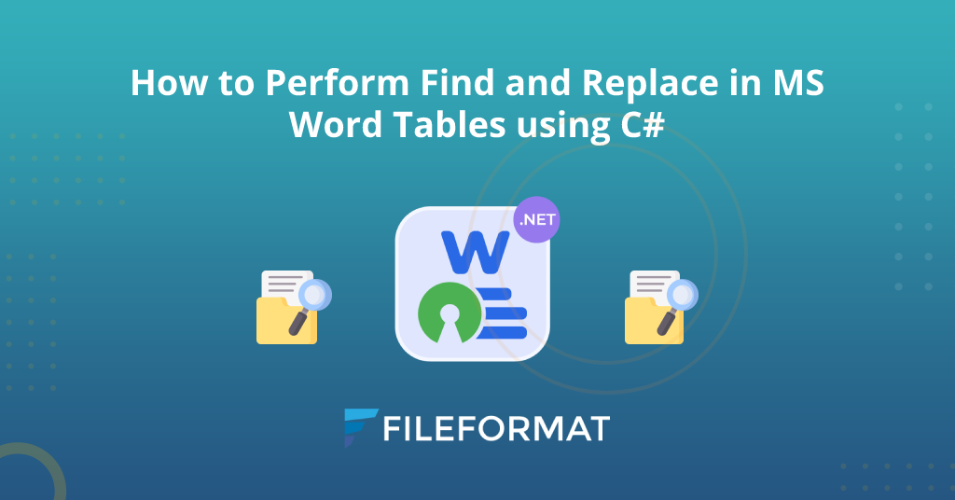
Overview
In a recent blog post, we discussed how to open a Word document using FileFormat.Words API. This article will cover how to perform find and replace in MS Word tables using C#. Before going forward, please also visit our blog post where you will discover how to programmatically create tables in Word files using FileFormat.Words. So, replacing a word in Word tables is a very common task and sometimes it becomes time-consuming in the case of large data tables. However, this open-source .NET library will help you make search and replace Word tables a lightweight process. Therefore, please go through this guide thoroughly to make sure that you have learned the process completely.
We will cover the following topics in this guide:
- Replace a word in Word files – API Installation
- How to replace text in Word documents programmatically
Replace a word in Word files – API Installation
The installation process of this open-source Word processing API is relatively straightforward. However, you can install FileFormat.Words by downloading the NuGet Package or running the following command in the NuGet Package manager.
Install-Package FileFormat.Words
How to replace text in Word documents programmatically?
Now, we can start writing code to perform find and replace in MS Word tables programmatically.
You may follow the steps and the code snippet mentioned below:
- Initialize an object of the Document class and load the Docx/Docs file.
- Instantiate an instance of the Body class.
- Create an object of the Table class.
- Invoke the FindTableByText method to find the number of occurrences of tables for the given text.
- Call the FindTableRow method to find a particular row along with the number of cells.
- Call the FindTableCell method to find a particular row along with the number of cells.
- Search and replace text in a Word document by calling the ChangeTextInCell method.
using FileFormat.Words;
using FileFormat.Words.Table;
namespace Example
{
class Program
{
static void Main(string[] args)
{
string path = "/Docs.docx";
// Initialize an object of the Document class and load the Docx/Docs file.
using (Document doc1 = new Document(path))
{
// Instantiate an instance of the Body class.
Body body1 = new Body(doc1);
// Create an object of the Table class.
Table table = new Table();
// Invoke the FindTableByText method to find the number of occurrences of tables for the given text.
int tableCount = body1.FindTableByText("British");
Console.WriteLine("number of tables with this text = " + tableCount);
// Call the FindTableRow method to find a particular row along with the number of cells.
foreach (TableRow row in body1.FindTableRow(0, 1))
{
Console.WriteLine(row.NumberOfCell);
}
// Call the FindTableRow method to find a particular row along with the number of cells.
foreach (TableCell cell in body1.FindTableCell(0, 1, 1))
{
Console.WriteLine(cell.Text);
Console.WriteLine(cell.CellWidth);
}
// Search and replace text in Word document by calling the ChangeTextInCell method.
Console.WriteLine(table.ChangeTextInCell(path, 0, 1, 2, "changed"));
}
}
}
}
Copy and paste the above code into your main file and run the program. Moreover, you can see the output in the image below:
![]() | ![]() |
Conclusion
This brings us to the end of this blog post. We hope you have learned how to perform find and replace in MS Word tables using FileFormat.Words library. Further, you have also gone through the methods offered by this open-source .NET API. In addition, there are other several methods to replace a word in Word documents. Therefore, do not forget to visit the documentation to learn about other methods and properties.
Finally, fileformat.com continues to write blog posts on other topics. Moreover, you can follow us on our social media platforms, including Facebook, LinkedIn, and Twitter.
Contribute
Since FileFormat.Words for .NET is an open-source project and is available on GitHub. So, the contribution from the community is much appreciated.
Ask a Question
You can let us know about your questions or queries on our forum.
Frequently Asked Questions – FAQs
How to find and replace in word using C#?
It is very easy to perform search and replace word in Word documents using an open-source .NET API. There are methods to create and edit tables programmatically.
How do I find and replace in a table in word?
Please visit this link to find the code snippet that helps you to replace text in Word tables using C#.