Siga esta publicación de blog para aprender cómo agregar encabezados de tabla en documentos de Word mediante programación. FileFormat.Words ofrece ricos métodos de creación y manipulación de tabla.
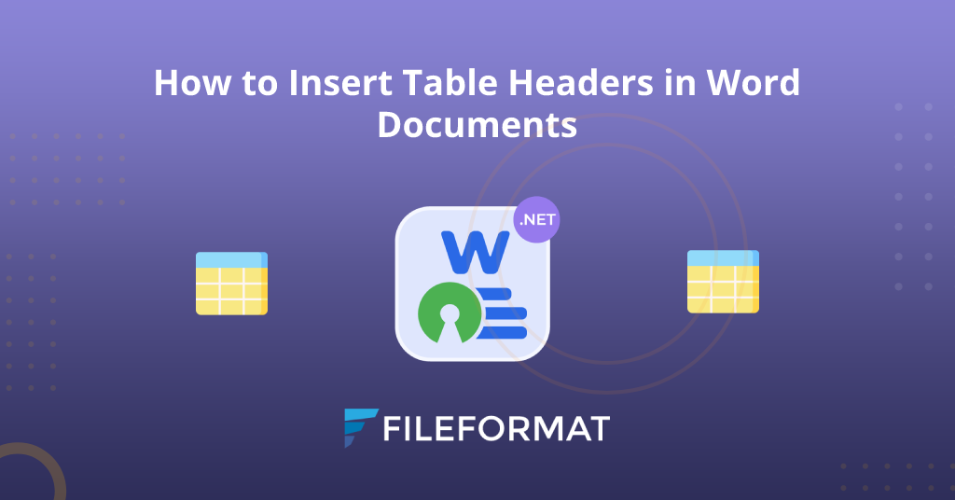
Descripción general
Las tablas de datos son elementos críticos en los documentos de MS Word. Trabajar con tablas es una tarea de rutina, pero ¿qué pasa si hay varios documentos con múltiples tablas de datos involucradas? Por supuesto, se necesitará algún tipo de automatización para automatizar las tareas repetitivas para ahorrar tiempo y aumentar la productividad. Por lo tanto, FileFormat.Words es una biblioteca .NET de código abierto para automatizar la creación, modificación y procesamiento de palabras. En este artículo, exploraremos cómo insertar encabezados de tabla en documentos de Word utilizando esta API C#. Sin embargo, puede visitar nuestros [artículos] anteriores 4 sobre varios temas relacionados con tablas en MS Word . Revisaremos las siguientes secciones en esta publicación de blog:
- Trabajando con encabezados de tabla - Instalación de API
- Agregar encabezados de tabla en archivos de Word mediante programación
Trabajar con encabezados de mesa - Instalación de API
El procedimiento de instalación de FileFormat.Words para la biblioteca .NET es cuestión de segundos. Esta API .NET de nivel empresarial proporciona una gran pila de características que los usuarios pueden aprovechar. Por lo tanto, puede descargar el paquete NUGET o ejecutar el siguiente comando en el Administrador de paquetes NUGET.
Install-Package FileFormat.Words
Agregar encabezados de tabla en archivos de Word mediante programación programada
La instalación se completa, el siguiente paso es escribir el fragmento de código de inmediato. Además, no solo podemos crear tabla en el documento de Word, sino que también podemos personalizar el diseño de tablas mediante programación. Puede seguir los pasos y el fragmento de código mencionado a continuación:
- Instanciar un objeto de la clase documento.
- Inicializar el constructor de la clase cuerpo con el objeto de clase de documento.
- Crear una instancia de la clase Tabla.
- Establezca el encabezado de la primera columna invocando el método TableHeaders.
- Invocar el método append para agregar las filas a la tabla.
- Llame al método AppendChild para agregar la tabla al cuerpo del documento.
- El método Guardar guardará el documento Word en el disco.
using FileFormat.Words;
using FileFormat.Words.Table;
namespace Example
{
class Program
{
static void Main(string[] args)
{
// Instantiate an object of the Document class.
using (Document doc = new Document())
{
// Initialize the constructor of the Body class with the Document class object.
Body body = new Body(doc);
// Create an instance of the Table class.
Table table = new Table();
// Initialize the constructor of the TopBorder class to set the border of the top side of the table.
TopBorder topBorder = new TopBorder();
// Invoke the dashed_border method to set the border style and border line width.
topBorder.dashed_border(20);
// To set the border of the bottom side of the table.
BottomBorder bottomBorder = new BottomBorder();
bottomBorder.dashed_border(20);
// To set the border of the right side of the table.
RightBorder rightBorder = new RightBorder();
rightBorder.dashed_border(20);
// To set the border of the left side of the table.
LeftBorder leftBorder = new LeftBorder();
leftBorder.dashed_border(20);
// To set the inside vertical border of the table.
InsideVerticalBorder insideVerticalBorder = new InsideVerticalBorder();
insideVerticalBorder.dashed_border(20);
// To set the inside vehorizontalrtical border of the table.
InsideHorizontalBorder insideHorizontalBorder = new InsideHorizontalBorder();
insideHorizontalBorder.dashed_border(20);
// Create an instance of the TableBorders class.
TableBorders tableBorders = new TableBorders();
// Append the object of the TopBorder class to the object of the TableBorders class.
tableBorders.AppendTopBorder(topBorder);
// Append the object of the BottomBorder class.
tableBorders.AppendBottomBorder(bottomBorder);
// Append the object of the RightBorder class.
tableBorders.AppendRightBorder(rightBorder);
// Append the object of the LeftBorder class.
tableBorders.AppendLeftBorder(leftBorder);
// Append the object of the InsideVerticalBorder class.
tableBorders.AppendInsideVerticalBorder(insideVerticalBorder);
// Append the object of the InsideHorizontalBorder class.
tableBorders.AppendInsideHorizontalBorder(insideHorizontalBorder);
// Initialize an instance of the TableProperties class.
TableProperties tblProp = new TableProperties();
// Invoke the Append method to attach the object of the TableBorders class.
tblProp.Append(tableBorders);
// Create an instance of the TableJustification class
TableJustification tableJustification = new TableJustification();
// Call the AlignLeft method to position the table on left side of the document.
tableJustification.AlignLeft();
// Invoke the Append method to attach the tableJustification object to the tblProp object.
tblProp.Append(tableJustification);
// The AppendChild method will attach the table propertiese to the table.
table.AppendChild(tblProp);
// Create an object of the TableRow class to create a table row.
TableRow tableRow = new TableRow();
TableRow tableRow2 = new TableRow();
// Initialize an istance of the TableCell class.
TableCell tableCell = new TableCell();
Paragraph para = new Paragraph();
Run run = new Run();
// Set the header of the first column by invoking the TableHeaders method.
table.TableHeaders("Country");
run.Text = "England";
para.AppendChild(run);
// Call the Append method to add text inside the table cell.
tableCell.Append(para);
// Create an object of the TableCellProperties table properties
TableCellProperties tblCellProps = new TableCellProperties();
// Set the width of table cell by initializing the object of the TableCellWidth class and append to tblCellProps object.
tblCellProps.Append(new TableCellWidth("2400"));
// Append method will attach the tblCellProps object with the object of the TableCell class.
tableCell.Append(tblCellProps);
TableCell tableCell2 = new TableCell();
Paragraph para2 = new Paragraph();
Run run2 = new Run();
// Invoke the TableHeaders method to set the header of the second column
table.TableHeaders("Capital");
run2.Text = "London";
para2.AppendChild(run2);
tableCell2.Append(para2);
TableCellProperties tblCellProps2 = new TableCellProperties();
tblCellProps2.Append(new TableCellWidth("1400"));
tableCell2.Append(tblCellProps2);
TableCell tableCell3 = new TableCell();
Paragraph para3 = new Paragraph();
Run run3 = new Run();
table.TableHeaders("Population");
run3.Text = "1000000";
para3.AppendChild(run3);
tableCell3.Append(para3);
TableCellProperties tblCellProps3 = new TableCellProperties();
tblCellProps3.Append(new TableCellWidth("1400"));
tableCell3.Append(tblCellProps3);
// Call the Append method to add cells into table row.
tableRow.Append(tableCell);
tableRow.Append(tableCell2);
tableRow.Append(tableCell3);
// create table cell
TableCell _tableCell = new TableCell();
Paragraph _para = new Paragraph();
Run _run = new Run();
_run.Text = "Pakistan";
_para.AppendChild(_run);
_tableCell.Append(_para);
TableCellProperties tblCellProps1_ = new TableCellProperties();
tblCellProps1_.Append(new TableCellWidth("2400"));
_tableCell.Append(tblCellProps1_);
TableCell _tableCell2 = new TableCell();
Paragraph _para2 = new Paragraph();
Run _run2 = new Run();
_run2.Text = "Islamabad";
_para2.AppendChild(_run2);
_tableCell2.Append(_para2);
TableCellProperties tblCellProps2_ = new TableCellProperties();
tblCellProps2_.Append(new TableCellWidth("1400"));
_tableCell2.Append(tblCellProps2_);
TableCell _tableCell3 = new TableCell();
Paragraph _para3 = new Paragraph();
Run _run3 = new Run();
_run3.Text = "2000000";
_para3.AppendChild(_run3);
_tableCell3.Append(_para3);
TableCellProperties tblCellProps3_ = new TableCellProperties();
tblCellProps3_.Append(new TableCellWidth("1400"));
_tableCell3.Append(tblCellProps3_);
tableRow2.Append(_tableCell);
tableRow2.Append(_tableCell2);
tableRow2.Append(_tableCell3);
// Invoke the Append method to add the rows into table.
table.Append(tableRow);
table.Append(tableRow2);
// Call the AppendChild method to add the table to the body of the document.
body.AppendChild(table);
// The Save method will save the Word document onto the disk.
doc.Save("/Users/Mustafa/Desktop/Docs.docx");
}
}
}
}
Copie y pegue el código anterior en su archivo principal y ejecute el programa. Verá la salida que se muestra en la imagen a continuación:
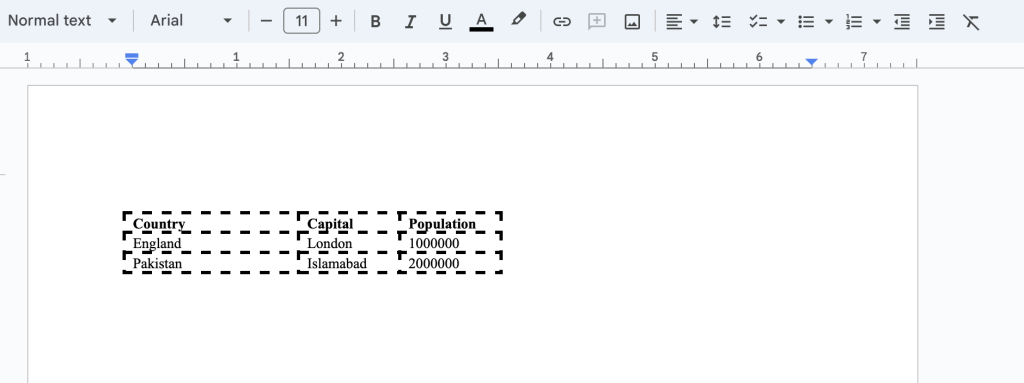
Conclusión
Estamos terminando esta publicación de blog aquí con la esperanza de haber aprendido cómo insertar encabezados de tabla en documentos de Word usando FileFormat.Words Biblioteca. Además, ha pasado por el proceso de instalación y el fragmento de código también. Además, existen otros métodos prácticos que puede explorar en la documentación. Finalmente, fileFormat.com continúa escribiendo publicaciones de blog sobre otros temas. Además, puede seguirnos en nuestras plataformas de redes sociales, incluidas Facebook, LinkedIn y Twitter.
Contribuir
Dado que FileFormat.Words para .NET es un proyecto de código abierto y está disponible en GitHub. Entonces, la contribución de la comunidad es muy apreciada.
Haga una pregunta
Puede informarnos sobre sus preguntas o consultas en nuestro Foro.
Preguntas frecuentes-Preguntas frecuentes
** ¿Cómo se inserta una tabla con encabezados?** Siga este enlace para aprender cómo insertar encabezados de tabla en C#.
Ver también
- Cómo crear un documento de Word en C# usando FileFormat.Words
- Cómo editar un documento de Word en C# usando FileFormat.Words
- Cómo hacer una tabla en archivos de Word usando fileformat.words
- Cómo realizar las tablas de búsqueda y reemplazar en MS usando C#
- ¿Cómo abro un archivo DOCX en C# usando FileFormat.Words?