این پست وبلاگ را دنبال کنید تا نحوه اضافه کردن عنوان های جدول در اسناد Word را به صورت برنامه ای اضافه کنید. FileFormat.Words روشهای غنی از ایجاد و روش های دستکاری را ارائه می دهد.
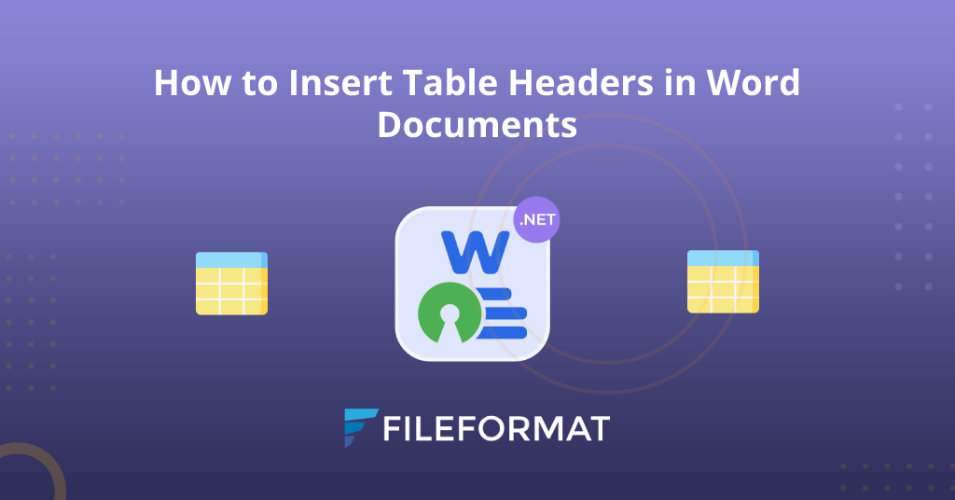
نمای کلی
جداول داده عناصر مهم در اسناد MS WORD است. کار با جداول یک کار معمول است اما اگر تعدادی از اسناد با جدول داده های متعدد وجود داشته باشد ، چه می شود؟ البته به نوعی اتوماسیون برای خودکارسازی وظایف تکراری برای صرفه جویی در وقت و تقویت بهره وری به شدت مورد نیاز خواهد بود. بنابراین ، FileFormat.words یک کتابخانه .NET با منبع باز برای خودکار سازی کلمه ، اصلاح و پردازش است. در این مقاله ، نحوه قرار دادن هدرهای جدول را در اسناد Word با استفاده از این C# API بررسی خواهیم کرد. با این حال ، می توانید [مقالات] قبلی ما را در موضوعات مختلف مربوط به جداول در MS Word بازدید کنید. ما در این پست وبلاگ بخش های زیر را طی خواهیم کرد:
کار با هدر جدول - نصب API
روش نصب FileFormat.Words برای کتابخانه .NET چند ثانیه است. این API سطح .NET در سطح شرکت ، تعداد گسترده ای از ویژگی هایی را که کاربران می توانند از آن استفاده کنند فراهم می کند. بنابراین ، می توانید بسته NUGET را بارگیری کنید یا دستور زیر را در مدیر بسته NUGET اجرا کنید.
Install-Package FileFormat.Words
اضافه کردن هدرهای جدول در پرونده های Word به صورت برنامه ای
نصب به پایان رسیده است ، مرحله بعدی نوشتن قطعه کد بلافاصله است. علاوه بر این ، ما نه تنها می توانیم جدول را در Word Document ایجاد کنیم بلکه می توانیم طرح جداول را بصورت برنامه ای سفارشی کنیم. ممکن است مراحل و قطعه کد ذکر شده در زیر را دنبال کنید:
- یک شیء از کلاس سند را فوری کنید.
- سازنده کلاس Body را با شیء کلاس Document آغاز کنید.
- نمونه ای از کلاس جدول ایجاد کنید.
- با استناد به روش TableHeaders هدر ستون اول را تنظیم کنید.
- از روش ضمیمه استفاده کنید تا ردیف ها را به جدول اضافه کنید.
- برای اضافه کردن جدول به بدنه سند ، با روش AppendChild تماس بگیرید.
- روش Save سند Word را روی دیسک ذخیره می کند.
using FileFormat.Words;
using FileFormat.Words.Table;
namespace Example
{
class Program
{
static void Main(string[] args)
{
// Instantiate an object of the Document class.
using (Document doc = new Document())
{
// Initialize the constructor of the Body class with the Document class object.
Body body = new Body(doc);
// Create an instance of the Table class.
Table table = new Table();
// Initialize the constructor of the TopBorder class to set the border of the top side of the table.
TopBorder topBorder = new TopBorder();
// Invoke the dashed_border method to set the border style and border line width.
topBorder.dashed_border(20);
// To set the border of the bottom side of the table.
BottomBorder bottomBorder = new BottomBorder();
bottomBorder.dashed_border(20);
// To set the border of the right side of the table.
RightBorder rightBorder = new RightBorder();
rightBorder.dashed_border(20);
// To set the border of the left side of the table.
LeftBorder leftBorder = new LeftBorder();
leftBorder.dashed_border(20);
// To set the inside vertical border of the table.
InsideVerticalBorder insideVerticalBorder = new InsideVerticalBorder();
insideVerticalBorder.dashed_border(20);
// To set the inside vehorizontalrtical border of the table.
InsideHorizontalBorder insideHorizontalBorder = new InsideHorizontalBorder();
insideHorizontalBorder.dashed_border(20);
// Create an instance of the TableBorders class.
TableBorders tableBorders = new TableBorders();
// Append the object of the TopBorder class to the object of the TableBorders class.
tableBorders.AppendTopBorder(topBorder);
// Append the object of the BottomBorder class.
tableBorders.AppendBottomBorder(bottomBorder);
// Append the object of the RightBorder class.
tableBorders.AppendRightBorder(rightBorder);
// Append the object of the LeftBorder class.
tableBorders.AppendLeftBorder(leftBorder);
// Append the object of the InsideVerticalBorder class.
tableBorders.AppendInsideVerticalBorder(insideVerticalBorder);
// Append the object of the InsideHorizontalBorder class.
tableBorders.AppendInsideHorizontalBorder(insideHorizontalBorder);
// Initialize an instance of the TableProperties class.
TableProperties tblProp = new TableProperties();
// Invoke the Append method to attach the object of the TableBorders class.
tblProp.Append(tableBorders);
// Create an instance of the TableJustification class
TableJustification tableJustification = new TableJustification();
// Call the AlignLeft method to position the table on left side of the document.
tableJustification.AlignLeft();
// Invoke the Append method to attach the tableJustification object to the tblProp object.
tblProp.Append(tableJustification);
// The AppendChild method will attach the table propertiese to the table.
table.AppendChild(tblProp);
// Create an object of the TableRow class to create a table row.
TableRow tableRow = new TableRow();
TableRow tableRow2 = new TableRow();
// Initialize an istance of the TableCell class.
TableCell tableCell = new TableCell();
Paragraph para = new Paragraph();
Run run = new Run();
// Set the header of the first column by invoking the TableHeaders method.
table.TableHeaders("Country");
run.Text = "England";
para.AppendChild(run);
// Call the Append method to add text inside the table cell.
tableCell.Append(para);
// Create an object of the TableCellProperties table properties
TableCellProperties tblCellProps = new TableCellProperties();
// Set the width of table cell by initializing the object of the TableCellWidth class and append to tblCellProps object.
tblCellProps.Append(new TableCellWidth("2400"));
// Append method will attach the tblCellProps object with the object of the TableCell class.
tableCell.Append(tblCellProps);
TableCell tableCell2 = new TableCell();
Paragraph para2 = new Paragraph();
Run run2 = new Run();
// Invoke the TableHeaders method to set the header of the second column
table.TableHeaders("Capital");
run2.Text = "London";
para2.AppendChild(run2);
tableCell2.Append(para2);
TableCellProperties tblCellProps2 = new TableCellProperties();
tblCellProps2.Append(new TableCellWidth("1400"));
tableCell2.Append(tblCellProps2);
TableCell tableCell3 = new TableCell();
Paragraph para3 = new Paragraph();
Run run3 = new Run();
table.TableHeaders("Population");
run3.Text = "1000000";
para3.AppendChild(run3);
tableCell3.Append(para3);
TableCellProperties tblCellProps3 = new TableCellProperties();
tblCellProps3.Append(new TableCellWidth("1400"));
tableCell3.Append(tblCellProps3);
// Call the Append method to add cells into table row.
tableRow.Append(tableCell);
tableRow.Append(tableCell2);
tableRow.Append(tableCell3);
// create table cell
TableCell _tableCell = new TableCell();
Paragraph _para = new Paragraph();
Run _run = new Run();
_run.Text = "Pakistan";
_para.AppendChild(_run);
_tableCell.Append(_para);
TableCellProperties tblCellProps1_ = new TableCellProperties();
tblCellProps1_.Append(new TableCellWidth("2400"));
_tableCell.Append(tblCellProps1_);
TableCell _tableCell2 = new TableCell();
Paragraph _para2 = new Paragraph();
Run _run2 = new Run();
_run2.Text = "Islamabad";
_para2.AppendChild(_run2);
_tableCell2.Append(_para2);
TableCellProperties tblCellProps2_ = new TableCellProperties();
tblCellProps2_.Append(new TableCellWidth("1400"));
_tableCell2.Append(tblCellProps2_);
TableCell _tableCell3 = new TableCell();
Paragraph _para3 = new Paragraph();
Run _run3 = new Run();
_run3.Text = "2000000";
_para3.AppendChild(_run3);
_tableCell3.Append(_para3);
TableCellProperties tblCellProps3_ = new TableCellProperties();
tblCellProps3_.Append(new TableCellWidth("1400"));
_tableCell3.Append(tblCellProps3_);
tableRow2.Append(_tableCell);
tableRow2.Append(_tableCell2);
tableRow2.Append(_tableCell3);
// Invoke the Append method to add the rows into table.
table.Append(tableRow);
table.Append(tableRow2);
// Call the AppendChild method to add the table to the body of the document.
body.AppendChild(table);
// The Save method will save the Word document onto the disk.
doc.Save("/Users/Mustafa/Desktop/Docs.docx");
}
}
}
}
کد فوق را در پرونده اصلی خود کپی و چسبانده و برنامه را اجرا کنید. خروجی نشان داده شده در تصویر زیر را مشاهده خواهید کرد:
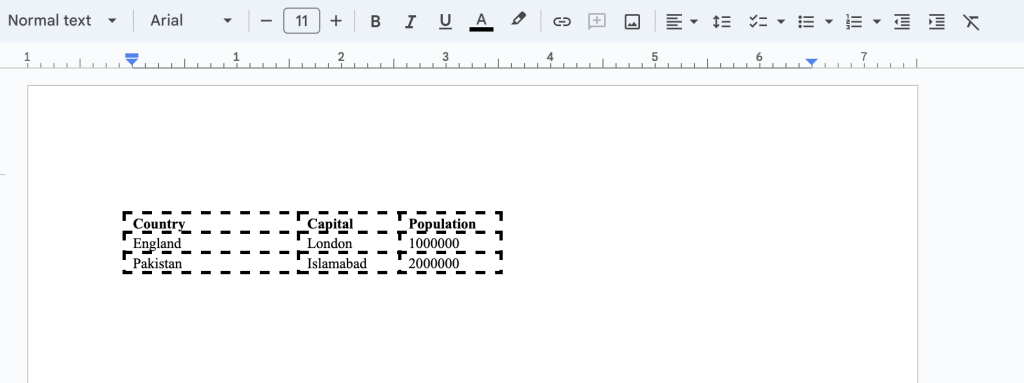
نتیجه گیری
ما این پست وبلاگ را در اینجا به پایان می رسانیم با این امید که شما یاد گرفته اید که چگونه می توانید عنوان های جدول را در اسناد Word با استفاده از کتابخانه FileFormat.Words وارد کنید. علاوه بر این ، شما روند نصب و قطعه کد را نیز طی کرده اید. علاوه بر این ، روشهای عملی دیگری نیز وجود دارد که می توانید در مستندات کشف کنید. سرانجام ، FileFormat.com به نوشتن پست های وبلاگ در موضوعات دیگر ادامه می دهد. علاوه بر این ، شما می توانید ما را در سیستم عامل های رسانه های اجتماعی ما ، از جمله فیس بوک ، LinkedIn و توییتر دنبال کنید.
مشارکت
از آنجا که FileFormat.Words for .NET یک پروژه منبع باز است و در GitHub در دسترس است. بنابراین ، از سهم جامعه بسیار استقبال می شود.
سوالی بپرسید.
شما می توانید در مورد سؤالات یا سؤالات خود در مورد [انجمن] ما به ما اطلاع دهید.
سوالات متداول-سؤالات متداول
** چگونه می توانید یک میز را با هدرها وارد کنید؟** لطفاً برای یادگیری نحوه درج هدرهای جدول در C#، این Link را دنبال کنید.
همچنین {.wp-block-heading} را ببینید
- نحوه ایجاد یک سند Word در C# با استفاده از FileFormat.Words
- نحوه ویرایش یک سند Word در C# با استفاده از FileFormat.Words
- نحوه تهیه جدول در پرونده های Word با استفاده از FileFormat.Words
- نحوه انجام یافتن و جایگزینی در جداول Word MS با استفاده از C#
- چگونه می توانم با استفاده از FileFormat.words ، یک پرونده Docx را در C# باز کنم؟