Enhance your document automation capabilities by leveraging the power of FileFormat.Words. Effortlessly add images to documents, taking your processing to new heights.
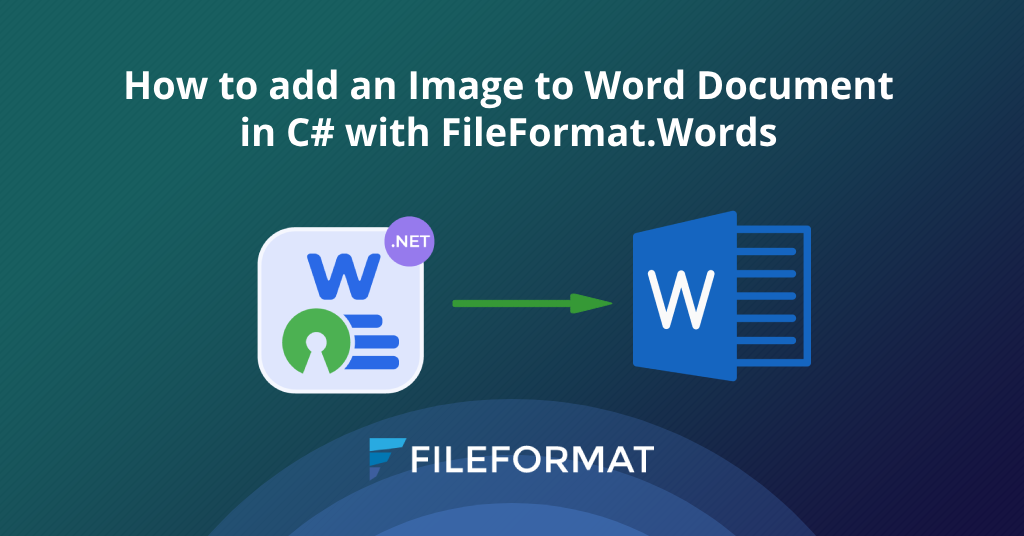
Overview
In today’s digital age, the use of images has become a fundamental aspect of document creation and presentation. Whether it’s a report, presentation, or any other form of written communication, images play a crucial role in enhancing visual appeal and conveying information more effectively. Microsoft Word, being one of the most widely used word processing tools, offers powerful features for incorporating images seamlessly into documents.
Enhance your document automation capabilities by leveraging the power of FileFormat.Words, a comprehensive document automation software that seamlessly integrates with MS Word. With FileFormat.Words, you can effortlessly incorporate images into your MS Word documents, taking your document processing to the next level.
In this blog post, we’ll explore inserting images into Word documents programmatically in C# using FileFormat.Words. We’ll cover the following sections
- ** Open Source API Installation:** Learn how to install the open source API for Word document automation.
- ** Adding an Image to a Word Document:** Discover the steps to programmatically insert images into Word documents using C#.
- ** Advanced Document Automation:** Explore advanced options and features provided by document automation software.
Open Source API Installation
Installing this open-source API in your .NET application is a breeze. FileFormat.Words is a lightweight library with powerful features for MS Word document manipulation. Simply download its NuGet Package or execute the following command in the NuGet Package Manager to effortlessly install this document automation software.
Install-Package FileFormat.Words
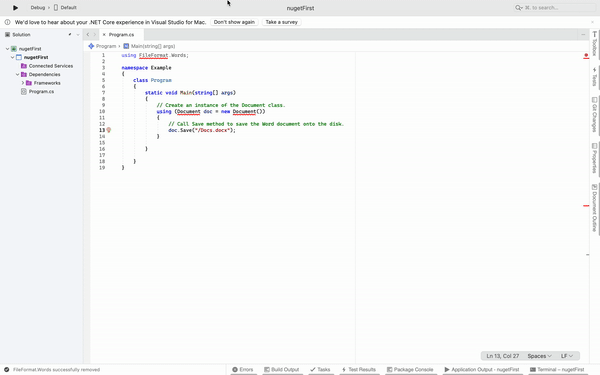
Adding an Image to a Word Document
Once you have installed this document automation software, you can proceed to write code snippets that showcase the process of programmatically creating and adding images to a Word document using C#. Below is an example code snippet that demonstrates how to create and add images to the document.
var documentPath = documentDir + "TestRunImage.docx";
var imagePath = imageDir + "testimage.jpeg";
var doc = new Document();
var body = new Body(doc);
var para = new Paragraph();
var run = new Run();
var image = new FileFormat.Words.Image(doc, imagePath, 100, 100);
run.AppendChild(image.Drawing);
para.AppendChild(run);
body.AppendChild(para);
doc.Save(documentPath);
The provided code snippet will generate a Word document and insert the image “testimage.jpg” into the document. The resulting output of the Word document can be observed in the image displayed below.
![Image added to word document][8]
Advanced Document Automation
With the help of this document automation software, we have accomplished the task of creating and adding images to a Word document. However, the functionality doesn’t end there. We can also extract images from existing Word documents. Let’s explore how to extract images from a document using the following code snippet.
using FileFormat.Words;
var doc = new Document("ImageDocument.docx");
var images = FileFormat.Words.Image.ExtractImagesFromDocument(doc);
// images is the List of stream that holds the extracted images stream
for (int i = 0; i < images.Count; i++)
{
using (FileStream fileStream = new FileStream($"extracted_image_{i}.jpg", FileMode.Create))
{
images[i].CopyTo(fileStream);
}
Conclusion
In this blog post, we explored the process of adding and extracting images from Word documents in C# using the powerful open-source .NET Library, FileFormat.Words for .NET. This enterprise-level API provides extensive features for programmatically creating and manipulating Word documents.
Comprehensive documentation is available to guide you in the development and utilization of this open-source Docx editor.
Stay connected with fileformat.com for future blog posts covering a wide range of topics. Follow us on social media platforms like Facebook, LinkedIn, and Twitter for updates and additional resources.
Contribute
Since FileFormat.Words for .NET is an open-source project and is available on GitHub. So, the contribution from the community is much appreciated.
Ask a Question
You can let us know about your questions or queries on our forum.
FAQs
How to insert an image to a word document using C#?
You may install FileFormat.Words for .NET in your .NET project to add images to Docx files programmatically.
How do I extract images from a Word document?
Please follow this link to learn how to extract images from Word documents using a C# library.