Install an open-source FileFormat.Words for .NET, learn how to read Docx file in C# programmatically. This API offers methods to build a Word file viewer.
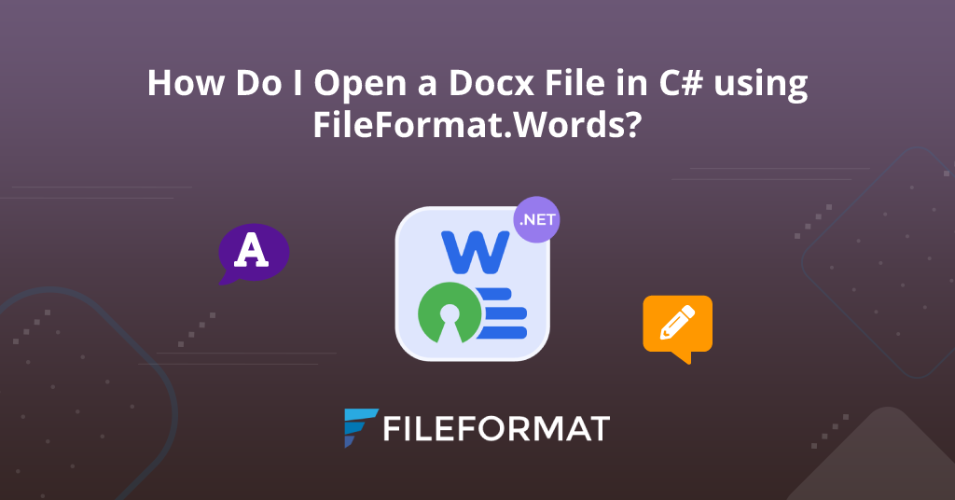
Overview
It is not easy to build business software that deals with file creation and manipulation immensely. Time and man hours are critical factors but opting for relevant third-party libraries plays a vital role in the development phase. Therefore, let’s go through an open-source .NET library that offers a wide range of methods to manipulate Word files programmatically. This API not only lets you create/edit Word files but you can also read business Docs/Docx files. We are talking about FileFormat.Words is a complete package of features necessary to process Word documents programmatically. However, this blog post will answer your question(i.e. How do I open a Docx file in C#?). Moreover, you will be able to build a Word file reader by the end of this article.
We will cover the following points in this guide:
Word File Viewer – API Installation
Please visit this link to go through the installation process in detail. Otherwise, it is quite easy to install, whether you can install it using its NuGet package or by running the following command in NuGet Package Manager.
Install-Package FileFormat.Words
How Do I Open a Docx file in C#
Once this open-source .NET API is installed, you can start writing the code right away. Let’s build a Word file reader component for your business software. There are multiple methods and properties to read a Docx/Docs file but we will use some prominent methods/properties.
You may follow the following steps and the code snippet:
- Initialize an instance of the Document class and load the Docx/Docs file.
- Instantiate an object of the Body class.
- Invoke the getDocumentTables.Count() method that returns the total number of tables in a document.
- The getDocumentTables property returns the table properties.
- The ExistingTableHeaders property returns the table headers.
- Call the NumberOfRows property to access the table rows.
- Use the NumberOfColumns property to fetch the number of columns.
- Access the number of cells using the NumberOfCells property.
- The TableBorder property is used to read the border style.
- Use the TablePosition property to get the position of the table.
- Invoke the ExtractImagesFromDocument method to get all the images from a Word document.
- Call the GetParagraphs method to retrieve all the document paragraphs.
- The LinesSpacing property is used to know the spacing between the lines.
- The Indent property is used to know the value of indentation.
- Get the text of the paragraph by calling the Text property.
using FileFormat.Words;
using FileFormat.Words.Table;
namespace Example
{
class Program
{
static void Main(string[] args)
{
// Initialize an instance of the Document class and load the Docx/Docs file.
using (Document doc1 = new Document("/Docs.docx"))
{
// Instantiate an object of the Body class.
Body body1 = new Body(doc1);
// Invoke the getDocumentTables method that returns the total number of tables in a document.
Console.WriteLine("Total Number of Tables " + body1.getDocumentTables.Count());
int i = 0;
// The getDocumentTables property returns the table properties.
foreach (Table props in body1.getDocumentTables)
{
// The ExistingTableHeaders property returns the table headers.
foreach (string tableHeader in props.ExistingTableHeaders)
{
i++;
Console.WriteLine("Header"+i+": "+tableHeader);
}
// Call NumberOfRows property to access the table rows.
Console.WriteLine("Number of rows "+props.NumberOfRows);
// Use NumberOfColumns property to fetch number of columns.
Console.WriteLine("Number of columns " + props.NumberOfColumns);
// Access the number of cells using NumberOfCells property.
Console.WriteLine("Number of cells " + props.NumberOfCells);
Console.WriteLine("Cell width " + props.CellWidth);
// The TableBorder property is used to read the border style.
Console.WriteLine("Border style " + props.TableBorder);
// Use the TablePosition property to get the position of the table.
Console.WriteLine("Table position " + props.TablePosition);
Console.WriteLine(" ");
}
// Invoke the ExtractImagesFromDocument method to get all the images from a Word document.
List<Stream> imageParts = Image.ExtractImagesFromDocument(doc1);
int imageCount = imageParts.Count;
Console.WriteLine($"Total number of images: {imageCount}");
// Call the GetParagraphs method to retrieve all the document paragraphs.
List<Paragraph> paras = body1.GetParagraphs();
Console.WriteLine("The number of Paragraphs " + paras.Count());
foreach (Paragraph p in paras)
{
// The LinesSpacing property is used to know the spacing between the lines.
Console.WriteLine("Line spacing "+p.LinesSpacing);
// The Indent property is used to know the value of indentation.
Console.WriteLine("Indent value "+p.Indent);
// Get the text of the paragraph by calling the Text property.
Console.WriteLine(p.Text);
}
}
}
}
}
The above code snippet reads a Word file that contains one paragraph, one table, and an image. However, you can see the output in the image below:
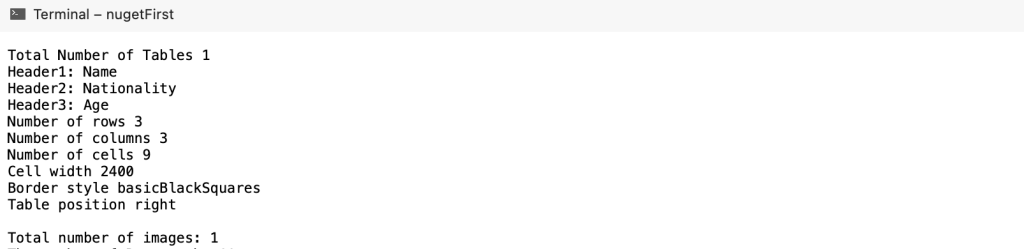
Conclusion
We are ending this blog post here. We hope that you have the answer to your question(i.e. How do I open a Docx file in C#?). In addition, we have gone through the code snippet that reads an existing Word document programmatically. Now, you can easily build a module that works like a Word file viewer. Further, do not forget to visit the documentation of FileFormat.Words for .NET.
Finally, fileformat.com continues to write blog posts on other topics. Moreover, you can follow us on our social media platforms, including Facebook, LinkedIn, and Twitter.
Contribute
Since FileFormat.Words for .NET is an open-source project and is available on GitHub. So, the contribution from the community is much appreciated.
Ask a Question
You can let us know about your questions or queries on our forum.
Frequently Asked Questions – FAQs
How do I open a DOCX file without Word?
You can open Docx/Docs files using this open-source .NET library FileFormat.Words. Moreover, you can build a Word file reader module for your business application using this library.