Forget the hassle and install FileFormat.Words to create & manipulate MS Word documents. This document automation software offers very useful features.
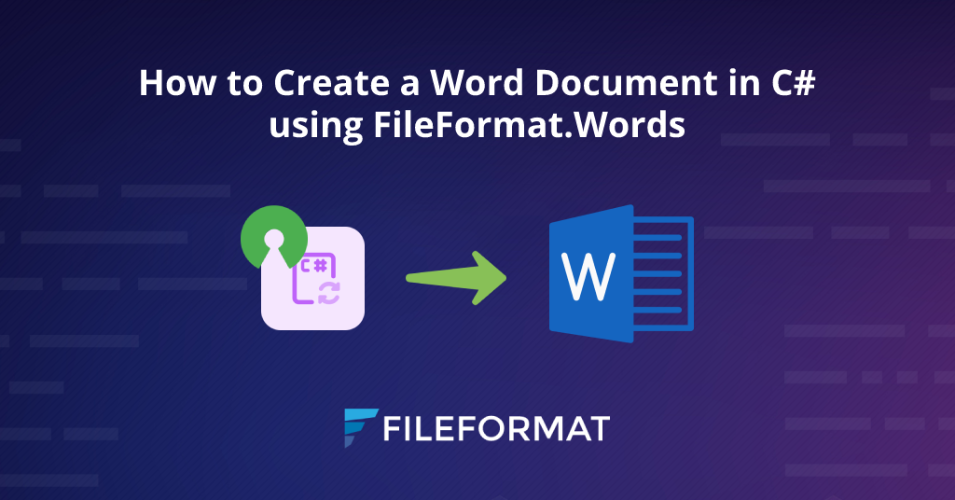
Overview
We are glad to announce the release of FileFormat.Words for .NET. This is an open-source API written in C# and enables .NET developers to create, design, and modify MS Word documents programmatically. No matter whether you are a beginner or an expert-level developer, you can easily integrate FileFormat.Words with your .NET application. In addition, no one can deny the significance and the usability of MS Word documents in any business. It is the most widely-used and multipurpose file format. In this blog post, we will learn how to create a Word document in C# and we will also write some code snippets to see FileFormat.Words for .NET in action.
We will cover the following sections:
- Word Document Generator – Open Source API Installation
- How to Create a Word Document Programmatically
- Document Automation Software – Advanced Options
Word Document Generator – Open Source API Installation
As far as the installation of this open-source API is concerned, it is easy to install and set up in your .NET application. In addition, FileFormat.Words library is lightweight and provides robust features to work with MS Word documents. So, download its NuGet Package or run the following command in the NuGet Package Manager to install this open-source document automation software.
Install-Package FileFormat.Words
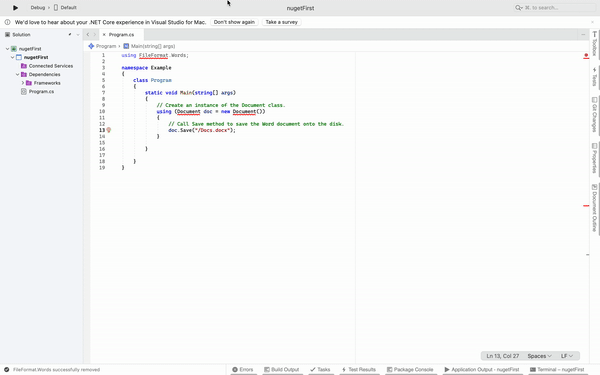
How to Create a Word Document Programmatically
Once this Word document generator API is installed, we can write code snippets to demonstrate how to create a Word document in C# programmatically.
You can follow the following steps to create an empty Word document:
- Initialize an instance of the Document class.
- Call the Save method to save the Word document onto the disk.
Copy and paste the following code snippet into your main file and run the program.
using FileFormat.Words;
namespace Example
{
class Program
{
static void Main(string[] args)
{
// Initialize an instance of the Document class.
Document doc = new Document();
// Call the Save method to save the Word document onto the disk.
doc.Save("/Docs.docx");
}
}
}
The above code snippet will generate an empty Word document as you can see the output in the image below:
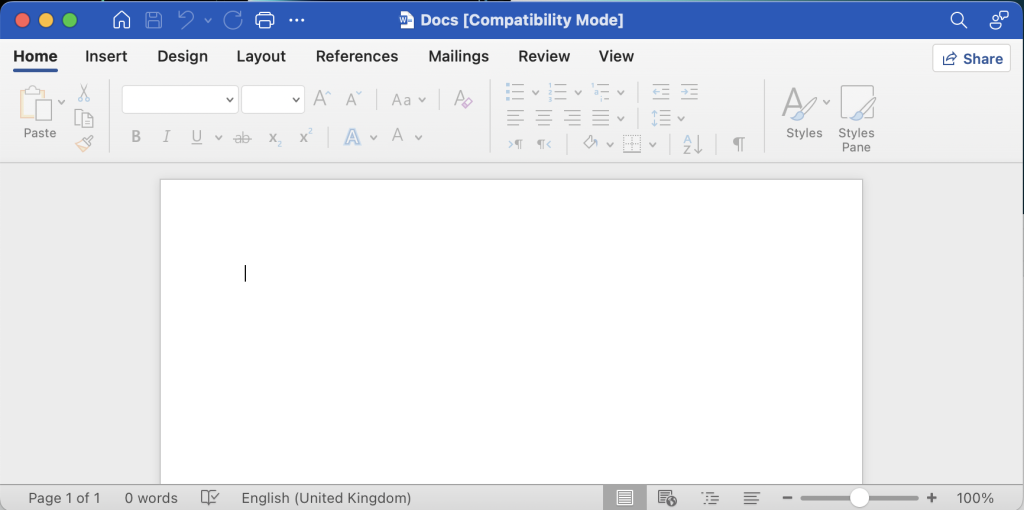
Document Automation Software – Advanced Options
We have successfully generated an empty Word document using this open-source Word document generator API. Now, we can not only add some text to a document but also style the text as per requirements. Let’s put a few lines of source code into our main file.
- Create an instance of the Document class.
- Initialize the constructor of the Body class with the Document class object.
- Instantiate an instance of the Paragraph class.
- Invoke the Text property to set the text of the paragraph.
- Likewise, call the Indent, LeftIndent, RihgtIndent, FirstLineIndent, Align, and LinesSpacing properties to format the paragraph.
- Call the AppendChild(paragraph) method to add the paragraph to the document.
- The Save method will save the Word document onto the disk.
using FileFormat.Words;
namespace Example
{
class Program
{
static void Main(string[] args)
{
// Create an instance of the Document class.
using (Document doc = new Document())
{
//Initialize the constructor of the Body class with the Document class object.
Body body = new Body(doc);
// Instantiate an instance of the Paragraph class.
Paragraph para1 = new Paragraph();
// Invoke the Text property to set the text of the paragraph.
para1.Text = "In publishing and graphic design, Lorem ipsum is a placeholder text commonly used to demonstrate the visual form of a document or a typeface without relying on meaningful content. Lorem ipsum may be used as a placeholder before final copy is available..";
para1.Indent = "300";
para1.LeftIndent = "250";
para1.RihgtIndent = "350";
para1.FirstLineIndent = "330";
para1.Align = "Left";
para1.LinesSpacing = "552";
// Call the AppendChild(paragraph) method to add the paragraph to the document.
body.AppendChild(para1);
// The Save method will save the Word document onto the disk.
doc.Save("/Docs.docx");
}
}
}
}
Update your main file with the above code snippet and run the project again. You will see the output generated by this open-source document automation software as shown in the image below:
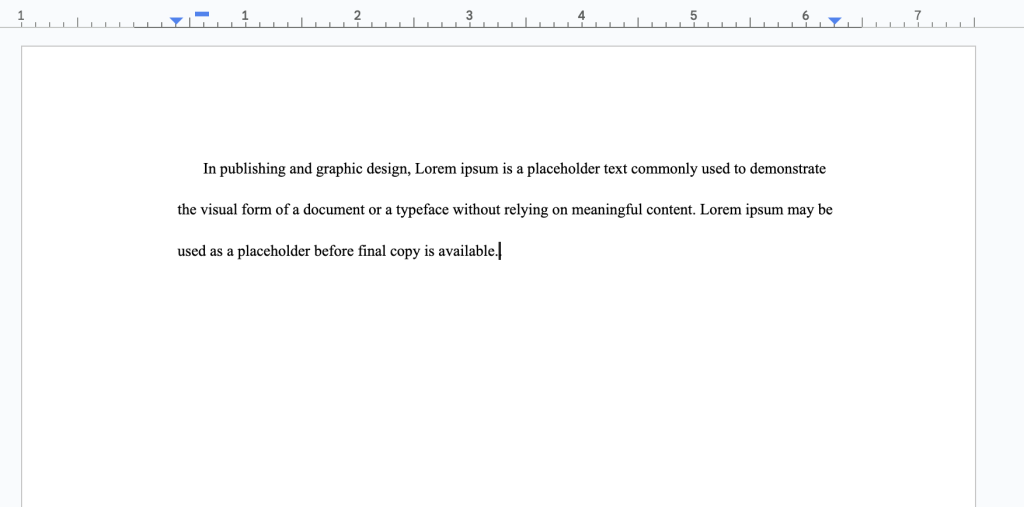
Conclusion
This brings us to the end of this blog post. In this guide, we learned how to create a Word document in C# using open-source FileFormat.Words. In addition, we have also gone through the installation procedure and some advanced features offered by this Word document generator library. Moreover, there are many other features that you can explore in the documentation.
Finally, fileformat.com is consistently writing tutorial blog posts on interesting topics. So, please stay in touch for regular updates. Moreover, you can follow us on our social media platforms, including Facebook, LinkedIn, and Twitter.
Contribute
Since FileFormat.Words for .NET is an open-source project and is available on GitHub. So, the contribution from the community is much appreciated.
Ask a Question
You can let us know about your questions or queries on our forum.
FAQs
How to create a new Word document in C#?
Please follow this link to learn Word document creation programmatically using this open-source document automation software FileFormat.Words.
How to create a Word document in .NET Core?
Download the Nuget Package of this open-source FileFormat.Words for .NET library that is written in C#. It is an enterprise-level .NET API developed for .NET developers if they plan to equip their business software with a Word document generator tool.