Install FileFormat.Words and edit Docx files programmatically. Word document processing is a matter of a few lines of source code with this open-source API.
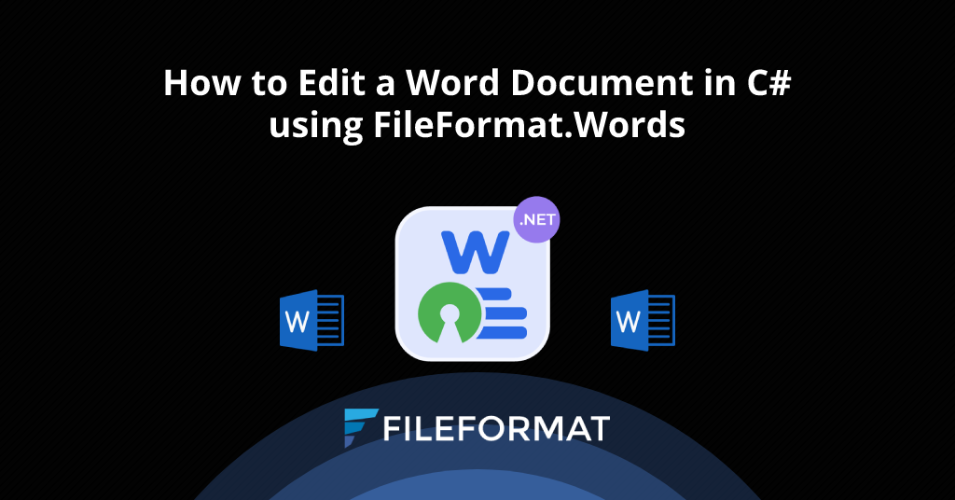
Overview
Welcome to another blog post in the continuation of FileFormat.Words for .NET exploration. In our previous article, we learned how to create a Word document in a .NET application using open-source FileFormat.Words. However, this open-source Docx editor lets you create Word documents and provides features to edit existing Word documents programmatically. In addition, this .NET library helps you build a document generator module for your business software. In this blog post, we will see how to edit a Word document in C# by installing FileFormat.Words into our .NET application project. Therefore, please go through this blog post thoroughly to learn the whole process which is quite easy and straight.
We will cover the following points in this article:
- Open-Source Docx Editor – API Installation
- How to Edit Docx File using FileFormat.Words
- How to Change Font in Word Document – Advanced Features
Open-Source Docx Editor – API Installation
The installation process of this open-source Docx editor is very simple as there are two ways to have this .NET library in your application project. However, you may download its NuGet Package or just run the following command in the NuGet Package Manager.
Install-Package FileFormat.Words
For more information about installation, please visit this link.
How to Edit Docx File using FileFormat.Words
This section demonstrates how to edit Docx file in C# using this open-source .NET library.
Please follow the following steps and the code snippet to achieve the functionality:
- Initialize an instance of the Document class and load an existing Word document.
- Instantiate the constructor of the Body class with the Document class object.
- Create an object of the Paragraph class.
- Instantiate an instance of the Run class that represents a run of characters in a Word document.
- Access the Text property of the Run class to set the text.
- Call the AppendChild method to attach the object of the Run class with the object of the Paragraph class.
- Invoke the AppendChild method of the body class to add a paragraph to the document.
- The Save method will save the Word document onto the disk.
using FileFormat.Words;
namespace Example
{
class Program
{
static void Main(string[] args)
{
// Initialize an instance of the Document class and load an existing Word document.
using (Document doc = new Document("/Docs.docx"))
{
//Instantiate the constructor of the Body class with the Document class object.
Body body = new Body(doc);
// Create an object of the Paragraph class.
Paragraph para = new Paragraph();
// Instantiate an instance of the Run class that represents a run of characters in a Word document.
Run run = new Run();
// Access the Text property of the Run class to set the text.
run.Text = "This is a sample text.";
// Call the AppendChild() method to attach the object of the Run class with the object of the Paragraph class.
para.AppendChild(run);
// Invoke AppendChild method of the body class to add paragraph to the document.
body.AppendChild(para);
// The Save method will save the Word document onto the disk.
doc.Save("/Docs.docx");
}
}
}
}
The output of the above code snippet is shown in the image below:
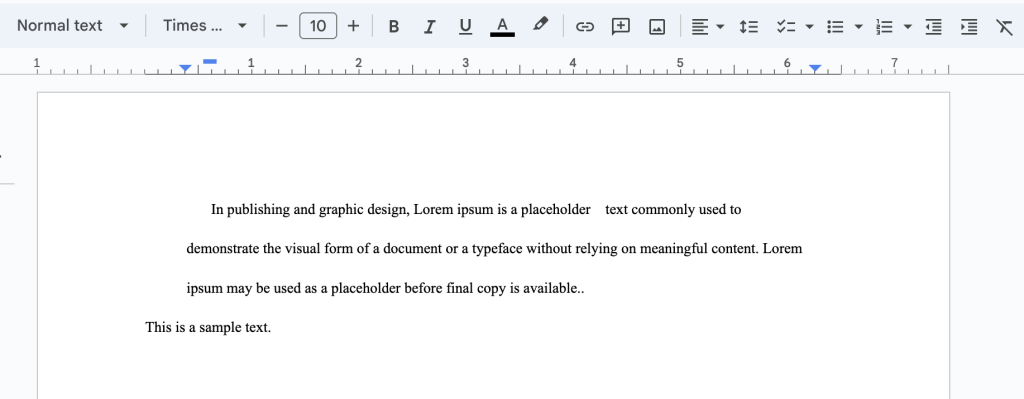
How to Change Font in Word Document – Advanced Features
FileFormat.Words also offers some advanced options to modify Word documents. Let’s see how can we edit Docx files further.
You may follow the following steps and the code snippet:
- Set the Bold property to true to make the text Bold.
- Make the Text Italic by setting the value of the Italic property.
- Set the value of the FontFamily property to set the font family of the Text.
- Access the FontSize property to set the font size.
- Set the Underline property to true to underline the text.
- The Color property will set the color of the text.
using FileFormat.Words;
namespace Example
{
class Program
{
static void Main(string[] args)
{
// Initialize an instance of the Document class and load an existing Word document.
using (Document doc = new Document("/Users/Mustafa/Desktop/Docs.docx"))
{
//Instantiate the constructor of the Body class with the Document class object.
Body body = new Body(doc);
// Create an object of the Paragraph class.
Paragraph para = new Paragraph();
// Instantiate an instance of the Run class that represents a run of characters in a Word document.
Run run = new Run();
// Access the Text property of the Run class to set the text.
run.Text = "This is a sample text.";
// Set the Bold property to true.
run.Bold = true;
// Make the Text Italic.
run.Italic = true;
// Set the value of FontFamily of the Text.
run.FontFamily = "Algerian";
// Access the FontSize property to set the font size.
run.FontSize = 40;
// Set the Underline property to true to underline the text.
run.Underline = true;
// The Color property will set the color of the text.
run.Color = "FF0000";
// Call the AppendChild() method to attach the object of the Run class with the object of the Paragraph class.
para.AppendChild(run);
// Invoke AppendChild method of the body class to add paragraph to the document.
body.AppendChild(para);
// The Save method will save the Word document onto the disk.
doc.Save("/Docs.docx");
}
}
}
}
The main file should look like the above code snippet. Please run the project and you will see the following output:
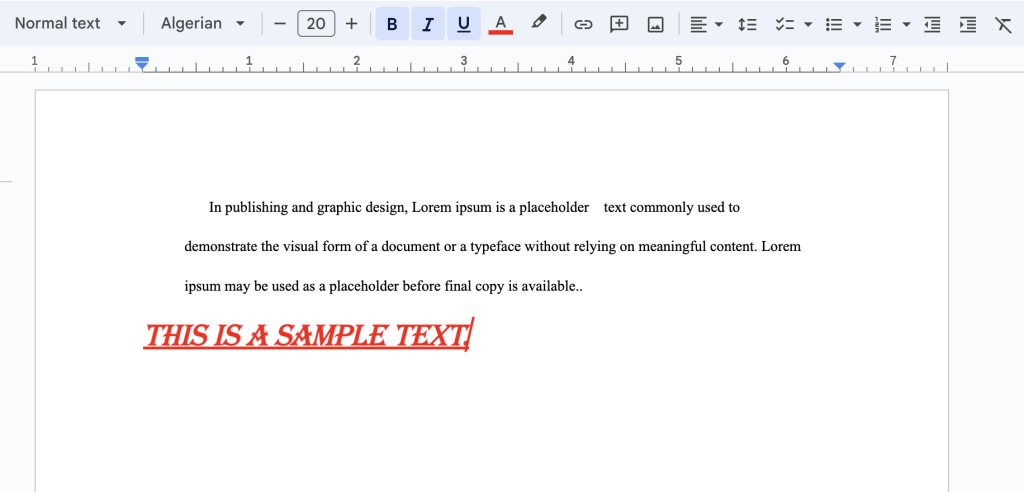
Conclusion
In this blog post, we learned how to edit a Word document in C# using an open-source .NET library. FileFormat.Words for .NET is an easy-to-use API that offers features to create and manipulate Word documents programmatically. In addition, we have also gone through how to change font in Word document along with other properties. Lastly, there is comprehensive documentation is available regarding the development and usage of this open-source Docx editor.
Finally, fileformat.com continues to write blog posts on other topics. So, please stay in touch for updates. Moreover, you can follow us on our social media platforms, including Facebook, LinkedIn, and Twitter.
Contribute
Since FileFormat.Words for .NET is an open-source project and is available on GitHub. So, the contribution from the community is much appreciated.
Ask a Question
You can let us know about your questions or queries on our forum.
FAQs
How to write in Word document using C#?
You may install FileFormat.Words for .NET in your .NET project to edit Docx files programmatically.
How do I completely edit a Word document?
Please follow this link to learn how to edit Word documents using a C# library.