Programmatic Word Document Formatting Made Simple. Learn how to format documents in Word programmatically using the powerful open-source API, FileFormat.Words.
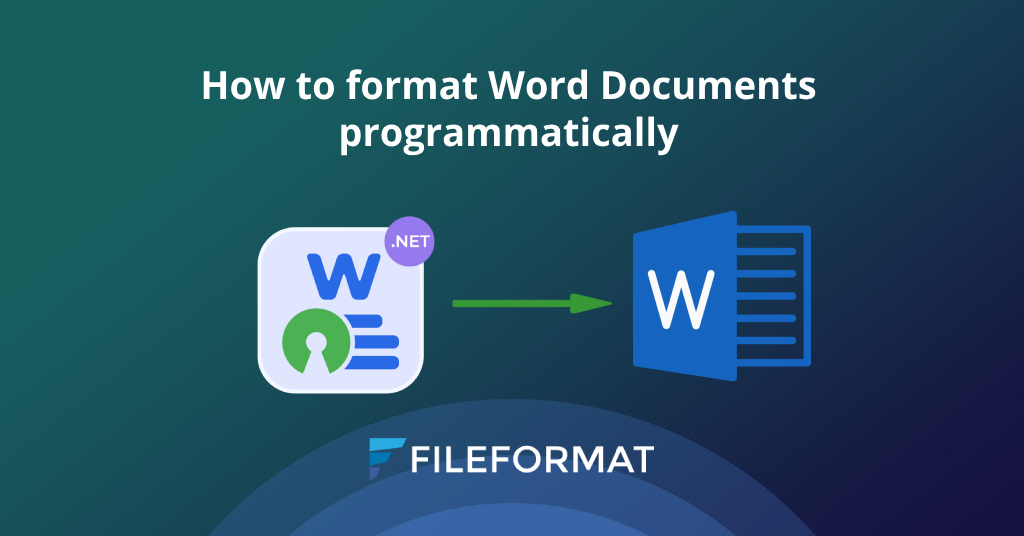
Overview
Welcome to another tutorial where we dive into the world of .NET with FileFormat.Words. In this article, we will focus on formatting Word documents programmatically using the capabilities of FileFormat.Words. With this comprehensive library, you can efficiently tailor the structure and style of your documents directly from your .NET applications. Let’s start our journey into programmatic document formatting!
This article covers the following topics:
- Installing the FileFormat.Words API
- How to Programmatically Format a Word Document using FileFormat.Words
Installing the FileFormat.Words API
The first step towards programmatic document formatting is to install FileFormat.Words into your project. You can easily add this open-source library via the NuGet Package Manager:
Install-Package FileFormat.Words
With this command, you’ll have the powerful .NET library at your disposal for formatting Word documents.
How to Programmatically Format a Word Document using FileFormat.Words
FileFormat.Words not only allows you to create and manipulate Word documents, but it also provides advanced formatting options for text within these documents. In the subsequent sections, we will delve deeper into how we can utilize these capabilities to enhance the presentation of our Docx files.
Here’s a breakdown of the process along with the corresponding code snippet:
- Use the Bold property and set it to true to embolden your text.
- Set the Italic property’s value to true to italicize your text.
- Employ the FontFamily property to change the font of your text.
- Adjust the text size with the FontSize property.
- To underline your text, simply set the Underline property to true.
- Alter the color of your text by manipulating the Color property.
using FileFormat.Words;
namespace Example
{
class Program
{
static void Main(string[] args)
{
// Initialize an instance of the Document class and load an existing Word document.
using (Document doc = new Document("testDocument.docx"))
{
// Instantiate the 'Body' class with the 'Document' class object.
Body documentBody = new Body(doc);
// Instantiate an object of the 'Paragraph' class.
Paragraph documentParagraph = new Paragraph();
// Instantiate an instance of the 'Run' class. It represents a run of characters in a Word document.
Run characterRun = new Run();
// Set the 'Text' property of the 'Run' class.
characterRun.Text = "This is a sample text.";
// Apply bold formatting to the text.
characterRun.Bold = true;
// Apply italic formatting to the text.
characterRun.Italic = true;
// Set the font of the text.
characterRun.FontFamily = "Algerian";
// Set the font size.
characterRun.FontSize = 40;
// Apply underline formatting to the text.
characterRun.Underline = true;
// Set the color of the text.
characterRun.Color = "FF0000";
// Use AppendChild() method to add the 'Run' class object to the 'Paragraph' class object.
documentParagraph.AppendChild(characterRun);
// Append the 'Paragraph' object to the 'Body' object.
documentBody.AppendChild(documentParagraph);
// Use 'Save' method to persist the Word document on the disk.
doc.Save("./testDocument.docx");
}
}
}
}
This example demonstrates how to apply text formatting in your document programmatically.
Conclusion
In this article, we walked through the process of programmatically formatting Word documents using the open-source library, FileFormat.Words for .NET. By leveraging this powerful API, we can effectively customize the appearance of our documents directly from our applications, improving efficiency and consistency in our workflows.
For more detailed guidance on using FileFormat.Words, check out the comprehensive documentation available.
Stay connected with us as we continue to explore the capabilities of FileFormat.Words in our future articles. Follow us on social media platforms such as Facebook, LinkedIn, and Twitter for the latest updates and insights.
Contribute
As FileFormat.Words for .NET is an open-source project hosted on GitHub, we strongly encourage and appreciate contributions from the community. Join us in our mission to simplify document formatting!
Questions?
You can post any questions or queries on our forum.