FileFormat.Words is an open-source library that offers a free Word processor module that programmatically lets you add/modify tables in Word documents.
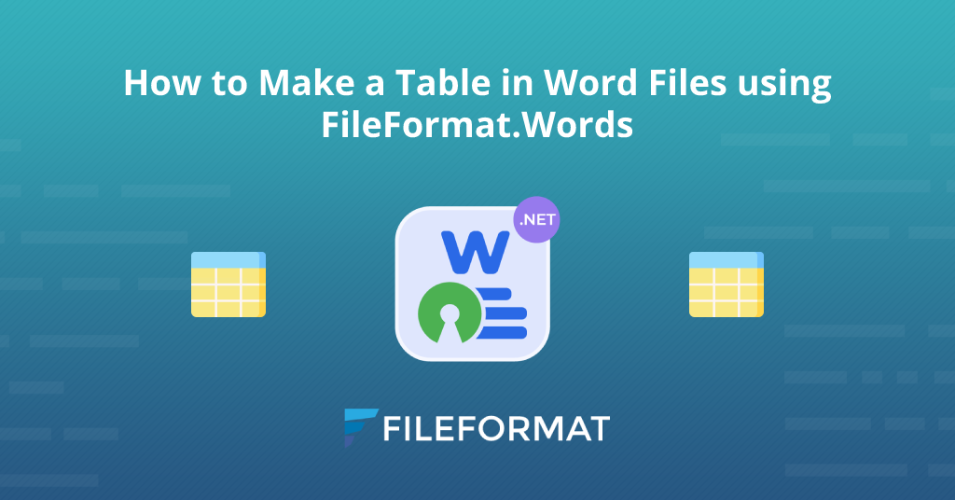
Overview
A Table in a Word document is considered an integral part when it comes to data representation. It is the most common document element and offers great convenience in terms of business document building. Amazingly, you can create a table in a Word document without using or installing MS Word on your local machine. Yes, let me introduce a free Word processor that enables you to create and manipulate Word documents programmatically. FileFormat.Words for .NET is a complete package of Word document processing. Therefore, in this blog post, we will learn how to make a table in Word documents using this .NET library FileFormat.Words.
This blog post covers the following sections:
- Table Generator for Word – API Installation
- How to Create a Table in a Word Document Programmatically
Table Generator for Word – API Installation
FileFormat.Words for .NET provides a wide range of features for MS Word processing. This open-source API is very easy to install. However, you can download its NuGet package of install it by running the following command into the NuGet Package manager.
Install-Package FileFormat.Words
How to Create a Table in a Word Document Programmatically
Let’s write some code to see this open-source free Word processor in action. In fact, we will see how to make a table in a Word document using FileFormat.Words library.
Please follow the following steps and the code snippet:
- Initialize an instance of the Document class.
- Instantiate the constructor of the Body class with the Document class object.
- Create an object of the Table class.
- Initialize the constructors of the TopBorder, BottomBorder, RightBorder, LeftBorder, InsideVerticalBorder, and InsideHorizontalBorder classes to set the border of all sides of the table.
- Invoke the basicBlackSquares_border method to set the border style and borderline width.
- Create an instance of the TableBorders class.
- Append the objects of the TopBorder, BottomBorder, RightBorder, LeftBorder, InsideVerticalBorder, and InsideHorizontalBorder classes to the object of the TableBorders class.
- Initialize an instance of the TableProperties class.
- Invoke the Append method of the TableProperties class to attach the object of the TableBorders class.
- Create an instance of the TableJustification class and call the AlignLeft method to position the table on the left side of the document.
- Invoke the Append method to attach the tableJustification object to the tblProp object.
- The AppendChild method of the Table class will attach the table properties to the table.
- Create an object of the TableRow class to create a table row.
- Initialize an instance of the TableCell class.
- Set the header of the first column by invoking the TableHeaders method.
- Call the Append method of the TableCell class to add text inside the table cell.
- Create an object of the TableCellProperties table properties
- Set the width of the table cell by initializing the object of the TableCellWidth class and append to tblCellProps object.
- The Append method will attach the tblCellProps object with the object of the TableCell class.
- Call the Append method to add the rows to the table.
- The AppendChild method will add the table to the body of the document.
- The Save method will save the Word document onto the disk.
using FileFormat.Words;
using FileFormat.Words.Table;
namespace Example
{
class Program
{
static void Main(string[] args)
{
// Initialize an instance of the Document class.
using (Document doc = new Document())
{
// Instantiate the constructor of the Body class with the Document class object.
Body body = new Body(doc);
// Create an object of the Table class.
Table table = new Table();
// Initialize the constructor of the TopBorder class to set the border of the top side of the table.
TopBorder topBorder = new TopBorder();
// Invoke the basicBlackSquares_border method to set the border style and border line width.
topBorder.basicBlackSquares_border(20);
// To set the border of the bottom side of the table.
BottomBorder bottomBorder = new BottomBorder();
bottomBorder.basicBlackSquares_border(20);
// To set the border of the right side of the table.
RightBorder rightBorder = new RightBorder();
rightBorder.basicBlackSquares_border(20);
// To set the border of the left side of the table.
LeftBorder leftBorder = new LeftBorder();
leftBorder.basicBlackSquares_border(20);
// To set the inside vertical border of the table.
InsideVerticalBorder insideVerticalBorder = new InsideVerticalBorder();
insideVerticalBorder.basicBlackSquares_border(20);
// To set the inside vehorizontalrtical border of the table.
InsideHorizontalBorder insideHorizontalBorder = new InsideHorizontalBorder();
insideHorizontalBorder.basicBlackSquares_border(20);
// Create an instance of the TableBorders class.
TableBorders tableBorders = new TableBorders();
// Append the object of the TopBorder class to the object of the TableBorders class.
tableBorders.AppendTopBorder(topBorder);
// Append the object of the BottomBorder class.
tableBorders.AppendBottomBorder(bottomBorder);
// Append the object of the RightBorder class.
tableBorders.AppendRightBorder(rightBorder);
// Append the object of the LeftBorder class.
tableBorders.AppendLeftBorder(leftBorder);
// Append the object of the InsideVerticalBorder class.
tableBorders.AppendInsideVerticalBorder(insideVerticalBorder);
// Append the object of the InsideHorizontalBorder class.
tableBorders.AppendInsideHorizontalBorder(insideHorizontalBorder);
// Initialize an instance of the TableProperties class.
TableProperties tblProp = new TableProperties();
// Invoke the Append method to attach the object of the TableBorders class.
tblProp.Append(tableBorders);
// Create an instance of the TableJustification class
TableJustification tableJustification = new TableJustification();
// Call the AlignLeft method to position the table on left side of the document.
tableJustification.AlignLeft();
// Invoke the Append method to attach the tableJustification object to the tblProp object.
tblProp.Append(tableJustification);
// The AppendChild method will attach the table propertiese to the table.
table.AppendChild(tblProp);
// Create an object of the TableRow class to create a table row.
TableRow tableRow = new TableRow();
TableRow tableRow2 = new TableRow();
// Initialize an istance of the TableCell class.
TableCell tableCell = new TableCell();
Paragraph para = new Paragraph();
Run run = new Run();
// Set the header of the first column by invoking the TableHeaders method.
table.TableHeaders("Name");
run.Text = "Mustafa";
para.AppendChild(run);
// Call the Append method to add text inside the table cell.
tableCell.Append(para);
// Create an object of the TableCellProperties table properties
TableCellProperties tblCellProps = new TableCellProperties();
// Set the width of table cell by initializing the object of the TableCellWidth class and append to tblCellProps object.
tblCellProps.Append(new TableCellWidth("2400"));
// Append method will attach the tblCellProps object with the object of the TableCell class.
tableCell.Append(tblCellProps);
TableCell tableCell2 = new TableCell();
Paragraph para2 = new Paragraph();
Run run2 = new Run();
// set the header of the second column
table.TableHeaders("Nationality");
run2.Text = "Pakistani";
para2.AppendChild(run2);
tableCell2.Append(para2);
TableCellProperties tblCellProps2 = new TableCellProperties();
tblCellProps2.Append(new TableCellWidth("1400"));
tableCell2.Append(tblCellProps2);
TableCell tableCell3 = new TableCell();
Paragraph para3 = new Paragraph();
Run run3 = new Run();
table.TableHeaders("Age");
run3.Text = "30";
para3.AppendChild(run3);
tableCell3.Append(para3);
TableCellProperties tblCellProps3 = new TableCellProperties();
tblCellProps3.Append(new TableCellWidth("1400"));
tableCell3.Append(tblCellProps3);
// Call the Append method to add cells into table row.
tableRow.Append(tableCell);
tableRow.Append(tableCell2);
tableRow.Append(tableCell3);
// create table cell
TableCell _tableCell = new TableCell();
Paragraph _para = new Paragraph();
Run _run = new Run();
_run.Text = "sultan";
_para.AppendChild(_run);
_tableCell.Append(_para);
TableCellProperties tblCellProps1_ = new TableCellProperties();
tblCellProps1_.Append(new TableCellWidth("2400"));
_tableCell.Append(tblCellProps1_);
TableCell _tableCell2 = new TableCell();
Paragraph _para2 = new Paragraph();
Run _run2 = new Run();
_run2.Text = "British";
_para2.AppendChild(_run2);
_tableCell2.Append(_para2);
TableCellProperties tblCellProps2_ = new TableCellProperties();
tblCellProps2_.Append(new TableCellWidth("1400"));
_tableCell2.Append(tblCellProps2_);
TableCell _tableCell3 = new TableCell();
Paragraph _para3 = new Paragraph();
Run _run3 = new Run();
_run3.Text = "2";
_para3.AppendChild(_run3);
_tableCell3.Append(_para3);
TableCellProperties tblCellProps3_ = new TableCellProperties();
tblCellProps3_.Append(new TableCellWidth("1400"));
_tableCell3.Append(tblCellProps3_);
tableRow2.Append(_tableCell);
tableRow2.Append(_tableCell2);
tableRow2.Append(_tableCell3);
// Call the Append method to add the rows into table.
table.Append(tableRow);
table.Append(tableRow2);
// The AppendChild method will add the table to the body of the document.
body.AppendChild(table);
// The Save method will save the Word document onto the disk.
doc.Save("/Docs.docx");
}
}
}
}
The output of the above code snippet is shown in the image below:
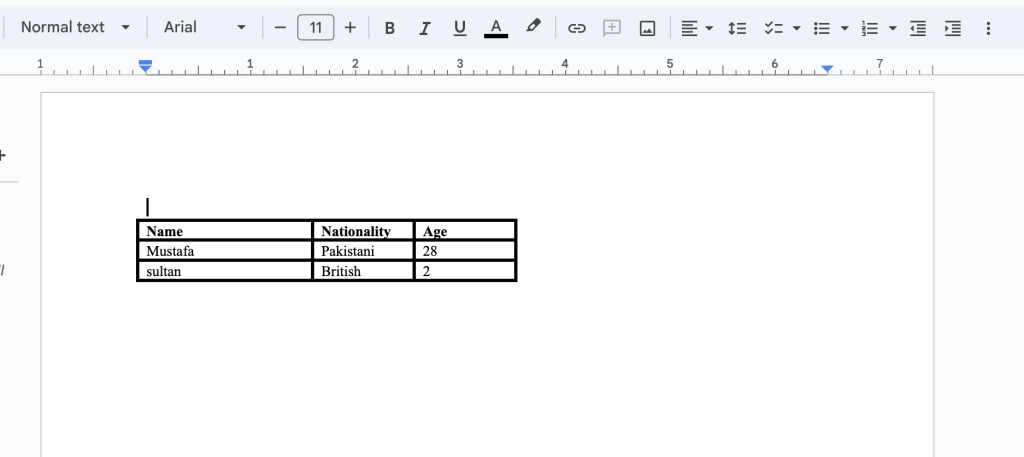
Conclusion
This brings us to the end of this article and we hope you have learned how to make a table in a Word document using FileFormat.Words library. In addition, this guide will help you if you are looking to build a table generator for Word documents in C#. Further, this free Word processor API is open source and you can find its documentation here.
Finally, fileformat.com continues to write blog posts on other topics. Moreover, you can follow us on our social media platforms, including Facebook, LinkedIn, and Twitter.
Contribute
Since FileFormat.Words for .NET is an open-source project and is available on GitHub. So, the contribution from the community is much appreciated.
Ask a Question
You can let us know about your questions or queries on our forum.
Frequently Asked Questions – FAQs
How do I create a table in a Word document?
It is very easy to create a table in Word document using this open-source .NET library. Moreover, you may explore this API further.
How to create a DOCX file in C#?
Please follow this link to go through a detailed code snippet and steps to create a Docx file in C#.
How do I create a custom table format in Word?
FileFormat.Words for .NET is a free library that offers features to manipulate and create MS Word programmatically. In fact, you may explore this namespace FileFormat.Words.Table to see the methods and properties.