Perform horizontal merge or vertical merge of one or more table cells in Docs/Docx files. FileFormat.Words provides methods to work with tables in Word files.
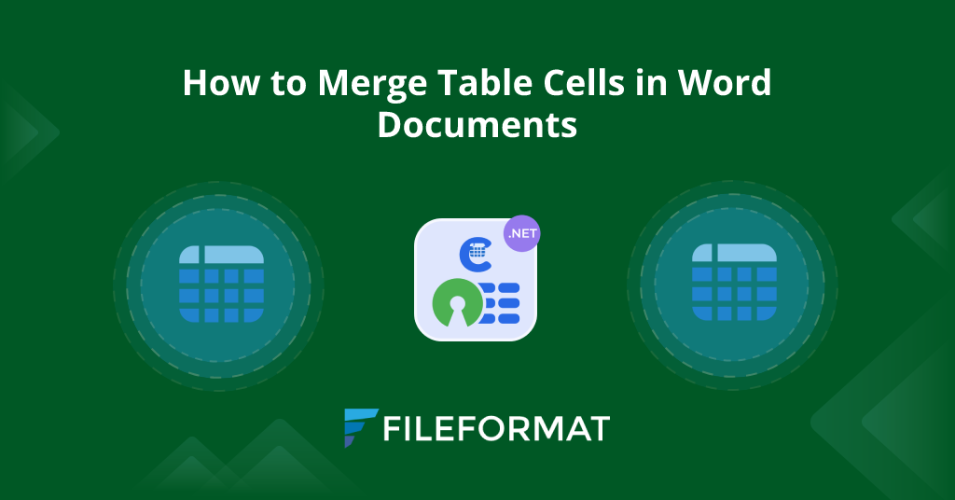
Overview
The newer version of FileFormat.Words offers further methods to work with tables in Word documents. The previous version contains methods to create, edit, and read table properties whereas the latest version lets users programmatically merge table cells in Docs/Docx files. In addition, you can do horizontal merge or vertical merge of table cells using this open-source .NET library. Moreover, it is an easy-to-use library whose methods are not complex and do not need any third-party dependency. In this blog post, we will learn how to merge table cells in Word documents. So, let’s start the installation process and start writing source code.
We will cover the following headings in this article:
Table Generator API Installation
Please visit this link for detailed information about the installation. Just to re-cap, the installation process of this free .NET API is relatively easy. Well, you can either download the NuGet package or run the following command in the NuGet Package Manager:
Install-Package FileFormat.Words
How to Merge Table Cells in Word Documents
We will write a code snippet to achieve horizontal merge and vertical merge of table cells. For that purpose, we will use the exposed classes and methods. Please visit this guide to learn how to create a table in a Word document using FileFormat.Words.
So, we will use further classes and methods in our code snippet:
- Create an object of the VerticalMerge class.
- MergeRestart property is used to specify that the element shall start a new vertically merged region in the table.
- Invoke the Append method to attach the verticalMerge object with the tblCellProps object.
- Instantiate an instance of the HorizontalMerge class.
- MergeRestart property is used to specify that the element shall start a new horizontally merged region in the table.
- Call the Append method to attach the horizontalMerge object with the tblCellProps object.
- MergeContinue property is used to specify that the element shall end a horizontally merged region in the table.
- MergeContinue property is used to specify that the element shall end a vertically merged region in the table.
using FileFormat.Words;
using FileFormat.Words.Table;
namespace Example
{
class Program
{
static void Main(string[] args)
{
// Initialize an instance of the Document class.
using (Document doc = new Document())
{
// Instantiate the constructor of the Body class with the Document class object.
Body body = new Body(doc);
// Create an object of the Table class.
Table table = new Table();
// Initialize the constructor of the TopBorder class to set the border of the top side of the table.
TopBorder topBorder = new TopBorder();
// Invoke the basicBlackSquares_border method to set the border style and border line width.
topBorder.basicBlackSquares_border(20);
// To set the border of the bottom side of the table.
BottomBorder bottomBorder = new BottomBorder();
bottomBorder.basicBlackSquares_border(20);
// To set the border of the right side of the table.
RightBorder rightBorder = new RightBorder();
rightBorder.basicBlackSquares_border(20);
// To set the border of the left side of the table.
LeftBorder leftBorder = new LeftBorder();
leftBorder.basicBlackSquares_border(20);
// To set the inside vertical border of the table.
InsideVerticalBorder insideVerticalBorder = new InsideVerticalBorder();
insideVerticalBorder.basicBlackSquares_border(20);
// To set the inside vehorizontalrtical border of the table.
InsideHorizontalBorder insideHorizontalBorder = new InsideHorizontalBorder();
insideHorizontalBorder.basicBlackSquares_border(20);
// Create an instance of the TableBorders class.
TableBorders tableBorders = new TableBorders();
// Append the object of the TopBorder class to the object of the TableBorders class.
tableBorders.AppendTopBorder(topBorder);
// Append the object of the BottomBorder class.
tableBorders.AppendBottomBorder(bottomBorder);
// Append the object of the RightBorder class.
tableBorders.AppendRightBorder(rightBorder);
// Append the object of the LeftBorder class.
tableBorders.AppendLeftBorder(leftBorder);
// Append the object of the InsideVerticalBorder class.
tableBorders.AppendInsideVerticalBorder(insideVerticalBorder);
// Append the object of the InsideHorizontalBorder class.
tableBorders.AppendInsideHorizontalBorder(insideHorizontalBorder);
// Initialize an instance of the TableProperties class.
TableProperties tblProp = new TableProperties();
// Invoke the Append method to attach the object of the TableBorders class.
tblProp.Append(tableBorders);
// Create an instance of the TableJustification class
TableJustification tableJustification = new TableJustification();
// Call the AlignLeft method to position the table on left side of the document.
tableJustification.AlignLeft();
// Invoke the Append method to attach the tableJustification object to the tblProp object.
tblProp.Append(tableJustification);
// The AppendChild method will attach the table properties to the table.
table.AppendChild(tblProp);
// Create an object of the TableRow class to create a table row.
TableRow tableRow = new TableRow();
TableRow tableRow2 = new TableRow();
// Initialize an instance of the TableCell class.
TableCell tableCell = new TableCell();
Paragraph para = new Paragraph();
Run run = new Run();
// Set the header of the first column by invoking the TableHeaders method.
table.TableHeaders("Name");
run.Text = "Mustafa";
para.AppendChild(run);
// Call the Append method to add text inside the table cell.
tableCell.Append(para);
// Create an object of the TableCellProperties table properties
TableCellProperties tblCellProps = new TableCellProperties();
// Set the width of table cell by initializing the object of the TableCellWidth class and append to tblCellProps object.
tblCellProps.Append(new TableCellWidth("2400"));
// Append method will attach the tblCellProps object with the object of the TableCell class.
tableCell.Append(tblCellProps);
TableCell tableCell2 = new TableCell();
Paragraph para2 = new Paragraph();
Run run2 = new Run();
// set the header of the second column
table.TableHeaders("Nationality");
run2.Text = "Pakistani";
para2.AppendChild(run2);
tableCell2.Append(para2);
TableCellProperties tblCellProps2 = new TableCellProperties();
// Create an object of the VerticalMerge class.
VerticalMerge verticalMerge = new VerticalMerge();
// MergeRestart property is used to specify that the element shall start a new vertically merged region in the table.
verticalMerge.MergeRestart = true;
// Invoke the Append method to attach the verticalMerge object with the tblCellProps object.
tblCellProps.Append(verticalMerge);
// Instantiate an instance of the HorizontalMerge class.
HorizontalMerge horizontalMerge = new HorizontalMerge();
// MergeRestart property is used to specify that the element shall start a new horizontally merged region in the table.
horizontalMerge.MergeRestart = true;
// Call the Append method to attach the horizontalMerge object with the tblCellProps object.
tblCellProps2.Append(horizontalMerge);
tblCellProps2.Append(new TableCellWidth("1400"));
tableCell2.Append(tblCellProps2);
TableCell tableCell3 = new TableCell();
Paragraph para3 = new Paragraph();
Run run3 = new Run();
table.TableHeaders("Age");
run3.Text = "30";
para3.AppendChild(run3);
tableCell3.Append(para3);
HorizontalMerge horizontalMerge1 = new HorizontalMerge();
// MergeContinue property is used to specify that the element shall end a horizontally merged region in the table.
horizontalMerge1.MergeContinue = true;
TableCellProperties tblCellProps3 = new TableCellProperties();
tblCellProps3.Append(new TableCellWidth("1400"));
tblCellProps3.Append(horizontalMerge1);
tableCell3.Append(tblCellProps3);
// Call the Append method to add cells into table row.
tableRow.Append(tableCell);
tableRow.Append(tableCell2);
tableRow.Append(tableCell3);
// create table cell
TableCell _tableCell = new TableCell();
Paragraph _para = new Paragraph();
Run _run = new Run();
_run.Text = "sultan";
_para.AppendChild(_run);
_tableCell.Append(_para);
TableCellProperties tblCellProps1_ = new TableCellProperties();
VerticalMerge verticalMerge2 = new VerticalMerge();
// MergeContinue property is used to specify that the element shall end a vertically merged region in the table.
verticalMerge2.MergeContinue = true;
tblCellProps1_.Append(verticalMerge2);
tblCellProps1_.Append(new TableCellWidth("2400"));
_tableCell.Append(tblCellProps1_);
TableCell _tableCell2 = new TableCell();
Paragraph _para2 = new Paragraph();
Run _run2 = new Run();
_run2.Text = "British";
_para2.AppendChild(_run2);
_tableCell2.Append(_para2);
TableCellProperties tblCellProps2_ = new TableCellProperties();
tblCellProps2_.Append(new TableCellWidth("1400"));
_tableCell2.Append(tblCellProps2_);
TableCell _tableCell3 = new TableCell();
Paragraph _para3 = new Paragraph();
Run _run3 = new Run();
_run3.Text = "2";
_para3.AppendChild(_run3);
_tableCell3.Append(_para3);
TableCellProperties tblCellProps3_ = new TableCellProperties();
tblCellProps3_.Append(new TableCellWidth("1400"));
_tableCell3.Append(tblCellProps3_);
tableRow2.Append(_tableCell);
tableRow2.Append(_tableCell2);
tableRow2.Append(_tableCell3);
// Call the Append method to add the rows into table.
table.Append(tableRow);
table.Append(tableRow2);
// The AppendChild method will add the table to the body of the document.
body.AppendChild(table);
// The Save method will save the Word document onto the disk.
doc.Save("/Users/Mustafa/Desktop/Docs.docx");
}
}
}
}
Copy and paste the above code segment into your main file and run. You will see the Word file generated with the content shown in the image below:
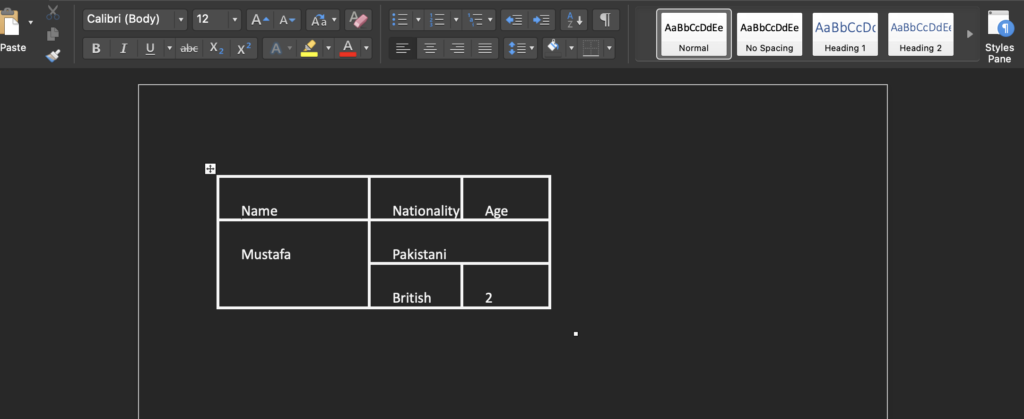
Conclusion
We are ending this blog post here with the hope that you have learned how to merge table cells in Word documents programmatically. In addition, we also have written the source code to implement horizontal merge and vertical merge of table cells. Therefore, you may opt for this open-source .NET table generator API to automate Word file automation. In the end, do not forget to visit the documentation to learn about further classes and methods.
Finally, fileformat.com is consistently writing tutorial blog posts on interesting topics. So, please stay in touch for regular updates. Moreover, you can follow us on our social media platforms, including Facebook, LinkedIn, and Twitter.
Contribute
Since FileFormat.Words for .NET is an open-source project and is available on GitHub. So, the contribution from the community is much appreciated.
Ask a Question
You can let us know about your questions or queries on our forum.
FAQs
How do I merge cells in a Word document?
You can merge table cells using VerticalMerge and HorizontalMerge classes.
How do I merge cells in a table in Doc?
Please follow this link to learn the steps and the code snippet to achieve this functionality.