Suivez cet article de blog pour apprendre à ajouter des en-têtes de table dans des documents de mots par programme. FileFormat.Words propose des méthodes de création et de manipulation de table riches.
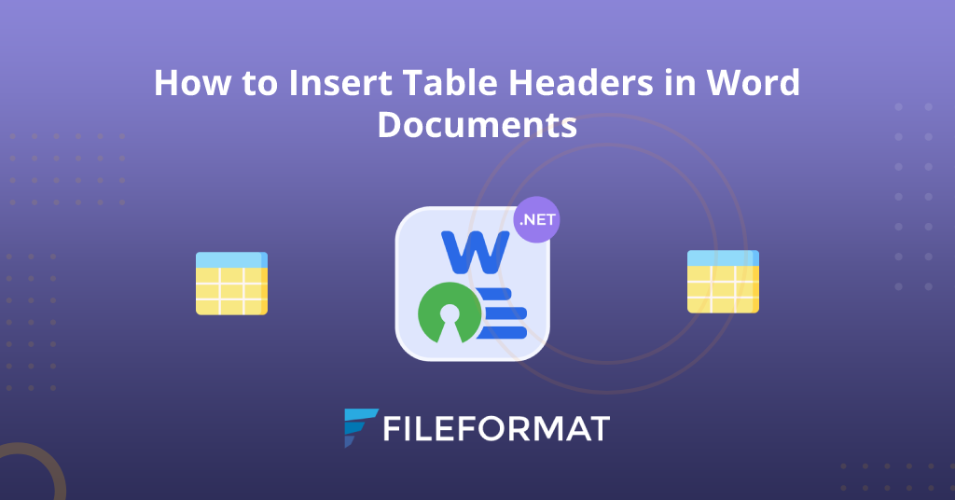
Présentation
Les tableaux de données sont des éléments critiques dans les documents MS word. Travailler avec des tables est une tâche de routine, mais que se passe-t-il s’il existe un certain nombre de documents avec plusieurs tables de données impliquées? Bien sûr, une sorte d’automatisation sera mal nécessaire pour automatiser les tâches répétitives pour gagner du temps et stimuler la productivité. Par conséquent, FileFormat.Words est une bibliothèque .NET open source pour automatiser la création, la modification et le traitement des mots. Dans cet article, nous explorerons comment insérer des en-têtes de table dans des documents Word en utilisant cette API C #. Cependant, vous pouvez visiter nos articles précédents sur divers sujets liés à tables dans MS Word . Nous passerons par les sections suivantes dans cet article de blog:
- Travailler avec des en-têtes de table - Installation de l’API
- Ajouter des en-têtes de table dans des fichiers Word programmatiquement
Travailler avec des en-têtes de table - Installation de l’API
La procédure d’installation de FileFormat.Words pour la bibliothèque .NET est une question de secondes. Cette API .NET au niveau de l’entreprise fournit une vaste pile de fonctionnalités que les utilisateurs peuvent exploiter. Ainsi, vous pouvez télécharger le Package NuGet ou exécuter la commande suivante dans le gestionnaire de package NuGet.
Install-Package FileFormat.Words
Ajout d’en-têtes de table dans des fichiers Word programmatiquement
L’installation est terminée, l’étape suivante consiste à écrire immédiatement l’extrait de code. De plus, nous pouvons non seulement créer une table dans un document Word, mais nous pouvons également personnaliser la mise en page des tables par programme. Vous pouvez suivre les étapes et l’extrait de code mentionné ci-dessous:
- Instancier un objet de la classe document.
- Initialisez le constructeur de la classe Body avec l’objet de classe de document.
- Créez une instance de la classe Table.
- Définissez l’en-tête de la première colonne en invoquant la méthode TableHeaders.
- Invoquez la méthode APPEND pour ajouter les lignes au tableau.
- Appelez la méthode appenchild pour ajouter le tableau au corps du document.
- La méthode Save enregistrera le document de mot sur le disque.
using FileFormat.Words;
using FileFormat.Words.Table;
namespace Example
{
class Program
{
static void Main(string[] args)
{
// Instantiate an object of the Document class.
using (Document doc = new Document())
{
// Initialize the constructor of the Body class with the Document class object.
Body body = new Body(doc);
// Create an instance of the Table class.
Table table = new Table();
// Initialize the constructor of the TopBorder class to set the border of the top side of the table.
TopBorder topBorder = new TopBorder();
// Invoke the dashed_border method to set the border style and border line width.
topBorder.dashed_border(20);
// To set the border of the bottom side of the table.
BottomBorder bottomBorder = new BottomBorder();
bottomBorder.dashed_border(20);
// To set the border of the right side of the table.
RightBorder rightBorder = new RightBorder();
rightBorder.dashed_border(20);
// To set the border of the left side of the table.
LeftBorder leftBorder = new LeftBorder();
leftBorder.dashed_border(20);
// To set the inside vertical border of the table.
InsideVerticalBorder insideVerticalBorder = new InsideVerticalBorder();
insideVerticalBorder.dashed_border(20);
// To set the inside vehorizontalrtical border of the table.
InsideHorizontalBorder insideHorizontalBorder = new InsideHorizontalBorder();
insideHorizontalBorder.dashed_border(20);
// Create an instance of the TableBorders class.
TableBorders tableBorders = new TableBorders();
// Append the object of the TopBorder class to the object of the TableBorders class.
tableBorders.AppendTopBorder(topBorder);
// Append the object of the BottomBorder class.
tableBorders.AppendBottomBorder(bottomBorder);
// Append the object of the RightBorder class.
tableBorders.AppendRightBorder(rightBorder);
// Append the object of the LeftBorder class.
tableBorders.AppendLeftBorder(leftBorder);
// Append the object of the InsideVerticalBorder class.
tableBorders.AppendInsideVerticalBorder(insideVerticalBorder);
// Append the object of the InsideHorizontalBorder class.
tableBorders.AppendInsideHorizontalBorder(insideHorizontalBorder);
// Initialize an instance of the TableProperties class.
TableProperties tblProp = new TableProperties();
// Invoke the Append method to attach the object of the TableBorders class.
tblProp.Append(tableBorders);
// Create an instance of the TableJustification class
TableJustification tableJustification = new TableJustification();
// Call the AlignLeft method to position the table on left side of the document.
tableJustification.AlignLeft();
// Invoke the Append method to attach the tableJustification object to the tblProp object.
tblProp.Append(tableJustification);
// The AppendChild method will attach the table propertiese to the table.
table.AppendChild(tblProp);
// Create an object of the TableRow class to create a table row.
TableRow tableRow = new TableRow();
TableRow tableRow2 = new TableRow();
// Initialize an istance of the TableCell class.
TableCell tableCell = new TableCell();
Paragraph para = new Paragraph();
Run run = new Run();
// Set the header of the first column by invoking the TableHeaders method.
table.TableHeaders("Country");
run.Text = "England";
para.AppendChild(run);
// Call the Append method to add text inside the table cell.
tableCell.Append(para);
// Create an object of the TableCellProperties table properties
TableCellProperties tblCellProps = new TableCellProperties();
// Set the width of table cell by initializing the object of the TableCellWidth class and append to tblCellProps object.
tblCellProps.Append(new TableCellWidth("2400"));
// Append method will attach the tblCellProps object with the object of the TableCell class.
tableCell.Append(tblCellProps);
TableCell tableCell2 = new TableCell();
Paragraph para2 = new Paragraph();
Run run2 = new Run();
// Invoke the TableHeaders method to set the header of the second column
table.TableHeaders("Capital");
run2.Text = "London";
para2.AppendChild(run2);
tableCell2.Append(para2);
TableCellProperties tblCellProps2 = new TableCellProperties();
tblCellProps2.Append(new TableCellWidth("1400"));
tableCell2.Append(tblCellProps2);
TableCell tableCell3 = new TableCell();
Paragraph para3 = new Paragraph();
Run run3 = new Run();
table.TableHeaders("Population");
run3.Text = "1000000";
para3.AppendChild(run3);
tableCell3.Append(para3);
TableCellProperties tblCellProps3 = new TableCellProperties();
tblCellProps3.Append(new TableCellWidth("1400"));
tableCell3.Append(tblCellProps3);
// Call the Append method to add cells into table row.
tableRow.Append(tableCell);
tableRow.Append(tableCell2);
tableRow.Append(tableCell3);
// create table cell
TableCell _tableCell = new TableCell();
Paragraph _para = new Paragraph();
Run _run = new Run();
_run.Text = "Pakistan";
_para.AppendChild(_run);
_tableCell.Append(_para);
TableCellProperties tblCellProps1_ = new TableCellProperties();
tblCellProps1_.Append(new TableCellWidth("2400"));
_tableCell.Append(tblCellProps1_);
TableCell _tableCell2 = new TableCell();
Paragraph _para2 = new Paragraph();
Run _run2 = new Run();
_run2.Text = "Islamabad";
_para2.AppendChild(_run2);
_tableCell2.Append(_para2);
TableCellProperties tblCellProps2_ = new TableCellProperties();
tblCellProps2_.Append(new TableCellWidth("1400"));
_tableCell2.Append(tblCellProps2_);
TableCell _tableCell3 = new TableCell();
Paragraph _para3 = new Paragraph();
Run _run3 = new Run();
_run3.Text = "2000000";
_para3.AppendChild(_run3);
_tableCell3.Append(_para3);
TableCellProperties tblCellProps3_ = new TableCellProperties();
tblCellProps3_.Append(new TableCellWidth("1400"));
_tableCell3.Append(tblCellProps3_);
tableRow2.Append(_tableCell);
tableRow2.Append(_tableCell2);
tableRow2.Append(_tableCell3);
// Invoke the Append method to add the rows into table.
table.Append(tableRow);
table.Append(tableRow2);
// Call the AppendChild method to add the table to the body of the document.
body.AppendChild(table);
// The Save method will save the Word document onto the disk.
doc.Save("/Users/Mustafa/Desktop/Docs.docx");
}
}
}
}
Copiez et collez le code ci-dessus dans votre fichier principal et exécutez le programme. Vous verrez la sortie indiquée dans l’image ci-dessous:
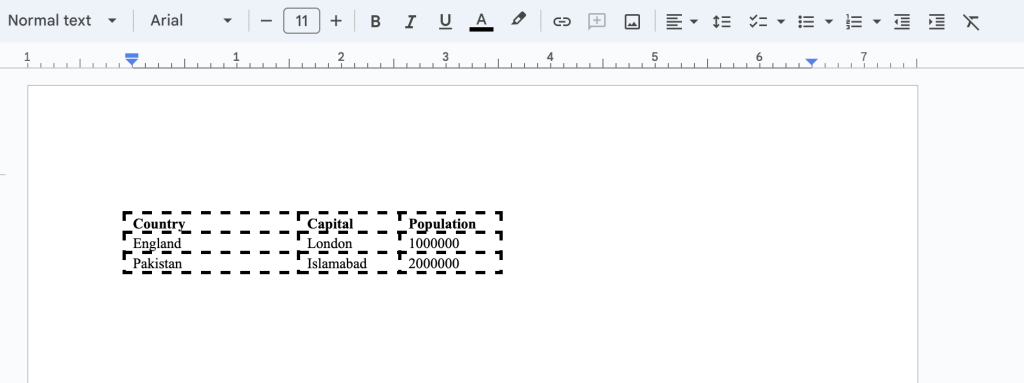
Conclusion
Nous terminons ce billet de blog ici dans l’espoir que vous ayez appris à insérer des en-têtes de table dans des documents Word à l’aide de la bibliothèque fileFormat.Words. De plus, vous avez également suivi le processus d’installation et l’extrait de code. De plus, il existe d’autres méthodes pratiques que vous pouvez explorer dans la documentation. Enfin, FileFormat.com continue d’écrire des articles de blog sur d’autres sujets. De plus, vous pouvez nous suivre sur nos plateformes de médias sociaux, notamment Facebook, LinkedIn et Twitter.
contribuer
Puisque FileFormat.Words pour .NET est un projet open-source et est disponible sur GitHub. Ainsi, la contribution de la communauté est très appréciée.
poser une question
Vous pouvez nous informer de vos questions ou questions sur notre Forum.
Questions fréquemment posées - FAQS
** Comment insérez-vous une table avec des en-têtes?** Veuillez suivre ce lien pour apprendre à insérer des en-têtes de table en C #.
Voir aussi
- Comment créer un document Word en C # à l’aide de fileformat.words
- Comment modifier un document Word en C # à l’aide de fileformat.words
- Comment faire une table dans des fichiers Word à l’aide de fileformat.words
- Comment effectuer la recherche et le remplacement dans les tables Word MS en utilisant C #
- Comment ouvrir un fichier docx en C # à l’aide de fileformat.words?