このブログ投稿に従って、Word Documentsにプログラムでテーブルヘッダーを追加する方法を学びます。 fileformat.wordsは、リッチテーブルの作成と操作方法を提供します。
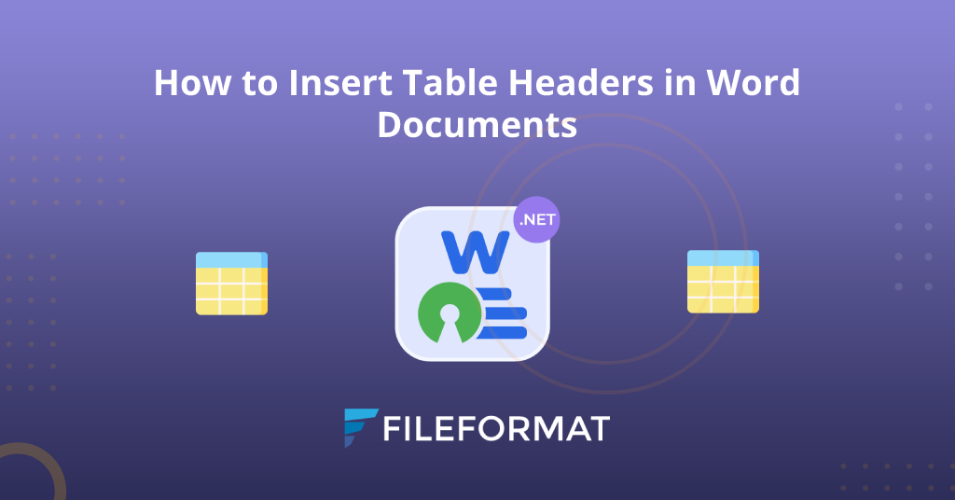
概要
データテーブルは、MS Wordドキュメントの重要な要素です。テーブルを操作することは日常的なタスクですが、複数のデータテーブルを含むドキュメントが多数ある場合はどうなりますか?もちろん、時間を節約して生産性を高めるために、繰り返しのタスクを自動化するために、ある種の自動化がひどく必要になります。したがって、fileformat.wordsは、単語の作成、変更、処理を自動化するためのオープンソース.NETライブラリです。この記事では、このC#APIを使用してWordドキュメントにテーブルヘッダーを挿入する方法について説明します。ただし、MS Word の テーブルに関連するさまざまなトピックについて、以前の記事にアクセスできます。 このブログ投稿の次のセクションについて説明します。
テーブルヘッダーの操作 - APIインストール
.NETライブラリのFileFormat.Wordsのインストール手順は数秒です。このエンタープライズレベルの.NET APIは、ユーザーが活用できる機能の膨大なスタックを提供します。したがって、nugetパッケージをダウンロードするか、Nugetパッケージマネージャーで次のコマンドを実行できます。
Install-Package FileFormat.Words
プログラムで単語ファイルのテーブルヘッダーを追加します
インストールが完了しました。次のステップは、コードスニペットをすぐに記述することです。さらに、Word Documentでテーブルを作成するだけでなく、テーブルのレイアウトをプログラムでカスタマイズすることもできます。 以下に説明する手順とコードスニペットに従うことができます。
- documentクラスのオブジェクトをインスタンス化します。
- ボディクラスのコンストラクターをドキュメントクラスオブジェクトで初期化します。
- 表クラスのインスタンスを作成します。
- Tableheadersメソッドを呼び出して、最初の列のヘッダーを設定します。
- append方法を呼び出して、テーブルに行を追加します。
- AppendChildメソッドを呼び出して、ドキュメントの本文にテーブルを追加します。
- saveメソッドは、wordドキュメントをディスクに保存します。
using FileFormat.Words;
using FileFormat.Words.Table;
namespace Example
{
class Program
{
static void Main(string[] args)
{
// Instantiate an object of the Document class.
using (Document doc = new Document())
{
// Initialize the constructor of the Body class with the Document class object.
Body body = new Body(doc);
// Create an instance of the Table class.
Table table = new Table();
// Initialize the constructor of the TopBorder class to set the border of the top side of the table.
TopBorder topBorder = new TopBorder();
// Invoke the dashed_border method to set the border style and border line width.
topBorder.dashed_border(20);
// To set the border of the bottom side of the table.
BottomBorder bottomBorder = new BottomBorder();
bottomBorder.dashed_border(20);
// To set the border of the right side of the table.
RightBorder rightBorder = new RightBorder();
rightBorder.dashed_border(20);
// To set the border of the left side of the table.
LeftBorder leftBorder = new LeftBorder();
leftBorder.dashed_border(20);
// To set the inside vertical border of the table.
InsideVerticalBorder insideVerticalBorder = new InsideVerticalBorder();
insideVerticalBorder.dashed_border(20);
// To set the inside vehorizontalrtical border of the table.
InsideHorizontalBorder insideHorizontalBorder = new InsideHorizontalBorder();
insideHorizontalBorder.dashed_border(20);
// Create an instance of the TableBorders class.
TableBorders tableBorders = new TableBorders();
// Append the object of the TopBorder class to the object of the TableBorders class.
tableBorders.AppendTopBorder(topBorder);
// Append the object of the BottomBorder class.
tableBorders.AppendBottomBorder(bottomBorder);
// Append the object of the RightBorder class.
tableBorders.AppendRightBorder(rightBorder);
// Append the object of the LeftBorder class.
tableBorders.AppendLeftBorder(leftBorder);
// Append the object of the InsideVerticalBorder class.
tableBorders.AppendInsideVerticalBorder(insideVerticalBorder);
// Append the object of the InsideHorizontalBorder class.
tableBorders.AppendInsideHorizontalBorder(insideHorizontalBorder);
// Initialize an instance of the TableProperties class.
TableProperties tblProp = new TableProperties();
// Invoke the Append method to attach the object of the TableBorders class.
tblProp.Append(tableBorders);
// Create an instance of the TableJustification class
TableJustification tableJustification = new TableJustification();
// Call the AlignLeft method to position the table on left side of the document.
tableJustification.AlignLeft();
// Invoke the Append method to attach the tableJustification object to the tblProp object.
tblProp.Append(tableJustification);
// The AppendChild method will attach the table propertiese to the table.
table.AppendChild(tblProp);
// Create an object of the TableRow class to create a table row.
TableRow tableRow = new TableRow();
TableRow tableRow2 = new TableRow();
// Initialize an istance of the TableCell class.
TableCell tableCell = new TableCell();
Paragraph para = new Paragraph();
Run run = new Run();
// Set the header of the first column by invoking the TableHeaders method.
table.TableHeaders("Country");
run.Text = "England";
para.AppendChild(run);
// Call the Append method to add text inside the table cell.
tableCell.Append(para);
// Create an object of the TableCellProperties table properties
TableCellProperties tblCellProps = new TableCellProperties();
// Set the width of table cell by initializing the object of the TableCellWidth class and append to tblCellProps object.
tblCellProps.Append(new TableCellWidth("2400"));
// Append method will attach the tblCellProps object with the object of the TableCell class.
tableCell.Append(tblCellProps);
TableCell tableCell2 = new TableCell();
Paragraph para2 = new Paragraph();
Run run2 = new Run();
// Invoke the TableHeaders method to set the header of the second column
table.TableHeaders("Capital");
run2.Text = "London";
para2.AppendChild(run2);
tableCell2.Append(para2);
TableCellProperties tblCellProps2 = new TableCellProperties();
tblCellProps2.Append(new TableCellWidth("1400"));
tableCell2.Append(tblCellProps2);
TableCell tableCell3 = new TableCell();
Paragraph para3 = new Paragraph();
Run run3 = new Run();
table.TableHeaders("Population");
run3.Text = "1000000";
para3.AppendChild(run3);
tableCell3.Append(para3);
TableCellProperties tblCellProps3 = new TableCellProperties();
tblCellProps3.Append(new TableCellWidth("1400"));
tableCell3.Append(tblCellProps3);
// Call the Append method to add cells into table row.
tableRow.Append(tableCell);
tableRow.Append(tableCell2);
tableRow.Append(tableCell3);
// create table cell
TableCell _tableCell = new TableCell();
Paragraph _para = new Paragraph();
Run _run = new Run();
_run.Text = "Pakistan";
_para.AppendChild(_run);
_tableCell.Append(_para);
TableCellProperties tblCellProps1_ = new TableCellProperties();
tblCellProps1_.Append(new TableCellWidth("2400"));
_tableCell.Append(tblCellProps1_);
TableCell _tableCell2 = new TableCell();
Paragraph _para2 = new Paragraph();
Run _run2 = new Run();
_run2.Text = "Islamabad";
_para2.AppendChild(_run2);
_tableCell2.Append(_para2);
TableCellProperties tblCellProps2_ = new TableCellProperties();
tblCellProps2_.Append(new TableCellWidth("1400"));
_tableCell2.Append(tblCellProps2_);
TableCell _tableCell3 = new TableCell();
Paragraph _para3 = new Paragraph();
Run _run3 = new Run();
_run3.Text = "2000000";
_para3.AppendChild(_run3);
_tableCell3.Append(_para3);
TableCellProperties tblCellProps3_ = new TableCellProperties();
tblCellProps3_.Append(new TableCellWidth("1400"));
_tableCell3.Append(tblCellProps3_);
tableRow2.Append(_tableCell);
tableRow2.Append(_tableCell2);
tableRow2.Append(_tableCell3);
// Invoke the Append method to add the rows into table.
table.Append(tableRow);
table.Append(tableRow2);
// Call the AppendChild method to add the table to the body of the document.
body.AppendChild(table);
// The Save method will save the Word document onto the disk.
doc.Save("/Users/Mustafa/Desktop/Docs.docx");
}
}
}
}
上記のコードをメインファイルにコピーして貼り付け、プログラムを実行します。以下の画像に示されている出力が表示されます。
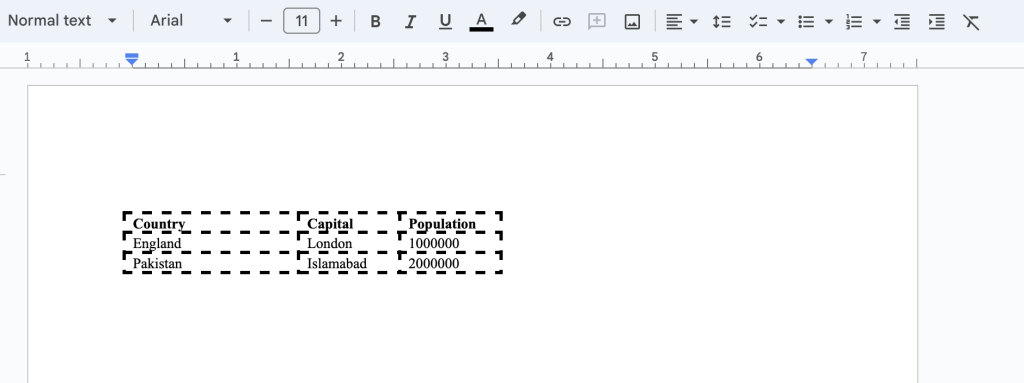
結論
このブログ投稿は、fileformat.wordsライブラリを使用して単語文書にテーブルヘッダーを挿入する方法を学んだことを期待して、ここで終了しています。さらに、インストールプロセスとコードスニペットも通過しました。さらに、ドキュメントで探索できる他の実用的な方法があります。 最後に、fileformat.comは、他のトピックに関するブログ投稿を書き続けています。さらに、Facebook、LinkedIn、Twitterなど、ソーシャルメディアプラットフォームでフォローできます。
貢献
.netのfileformat.wordsはオープンソースプロジェクトであり、githubで入手可能です。したがって、コミュニティからの貢献は大歓迎です。
質問する
フォーラムでの質問や質問についてお知らせください。
よくある質問 - FAQS
ヘッダー付きのテーブルをどのように挿入しますか? このリンクに従って、C#にテーブルヘッダーを挿入する方法を学習してください。