fileformat.wordsをインストールし、プログラムでdocxファイルを編集します。 Word Document処理は、このオープンソースAPIを備えた数行のソースコードの問題です。
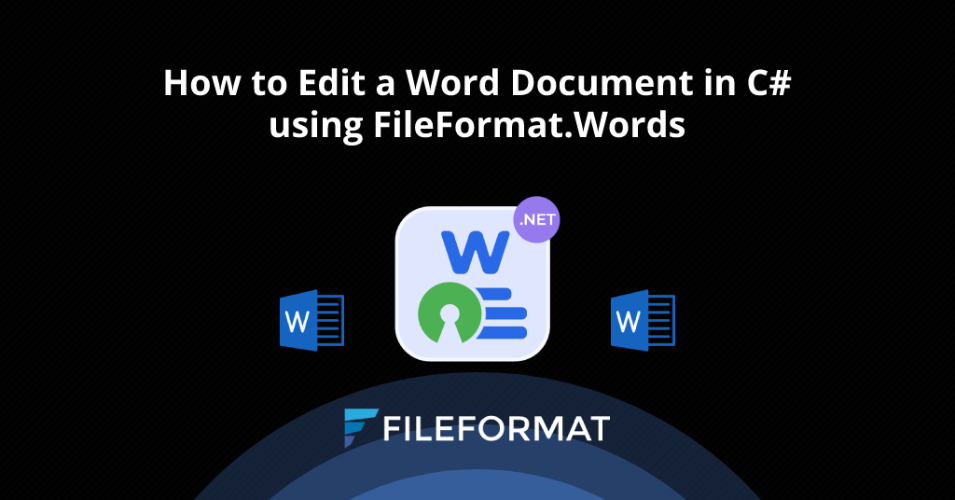
概要
.netのfileformat.words探査の継続における別のブログ投稿へようこそ。以前の記事で、オープンソースFileFormat.Wordsを使用して.NETアプリケーションでWordドキュメントを作成する方法を学びました。ただし、この OpenSource Docxエディター を使用すると、Wordドキュメントを作成し、既存のWordドキュメントをプログラムで編集する機能を提供できます。さらに、この.NETライブラリは、ビジネスソフトウェア向けのドキュメントジェネレーターモジュールを構築するのに役立ちます。このブログ投稿では、fileformat.wordsを.NETアプリケーションプロジェクトにインストールして、C#**でWord Documentを編集する方法を確認します。したがって、このブログ投稿を徹底的に進めて、非常に簡単でまっすぐなプロセス全体を学習してください。 この記事では、次のポイントについて説明します。
オープンソースDOCXエディター - APIインストール
この オープンソースDocxエディター のインストールプロセスは、アプリケーションプロジェクトにこの.NETライブラリを使用する方法が2つあるため、非常に簡単です。ただし、nugetパッケージをダウンロードするか、Nugetパッケージマネージャーで次のコマンドを実行するだけです。
Install-Package FileFormat.Words
インストールの詳細については、このリンクをご覧ください。
fileformat.wordsを使用してdocxファイルを編集する方法
このセクションでは、このオープンソース.NETライブラリを使用して、c# でdocxファイルを 編集する方法を示します。 次の手順とコードスニペットに従って、機能を実現してください。
- Documentクラスのインスタンスを初期化し、既存のWordドキュメントをロードします。
- ボディクラスのコンストラクターをドキュメントクラスオブジェクトにインスタンス化します。
- 段落クラスのオブジェクトを作成します。
- Wordドキュメントの文字の実行を表す実行クラスのインスタンスをインスタンスにします。
- テキスト実行クラスのプロパティにアクセスして、テキストを設定します。
- AppendChildメソッドを呼び出して、実行クラスのオブジェクトを段落クラスのオブジェクトに接続します。
- ボディクラスのappendChild方法を呼び出して、ドキュメントに段落を追加します。
- saveメソッドは、wordドキュメントをディスクに保存します。
using FileFormat.Words;
namespace Example
{
class Program
{
static void Main(string[] args)
{
// Initialize an instance of the Document class and load an existing Word document.
using (Document doc = new Document("/Docs.docx"))
{
//Instantiate the constructor of the Body class with the Document class object.
Body body = new Body(doc);
// Create an object of the Paragraph class.
Paragraph para = new Paragraph();
// Instantiate an instance of the Run class that represents a run of characters in a Word document.
Run run = new Run();
// Access the Text property of the Run class to set the text.
run.Text = "This is a sample text.";
// Call the AppendChild() method to attach the object of the Run class with the object of the Paragraph class.
para.AppendChild(run);
// Invoke AppendChild method of the body class to add paragraph to the document.
body.AppendChild(para);
// The Save method will save the Word document onto the disk.
doc.Save("/Docs.docx");
}
}
}
}
上記のコードスニペットの出力は、以下の画像に示されています。
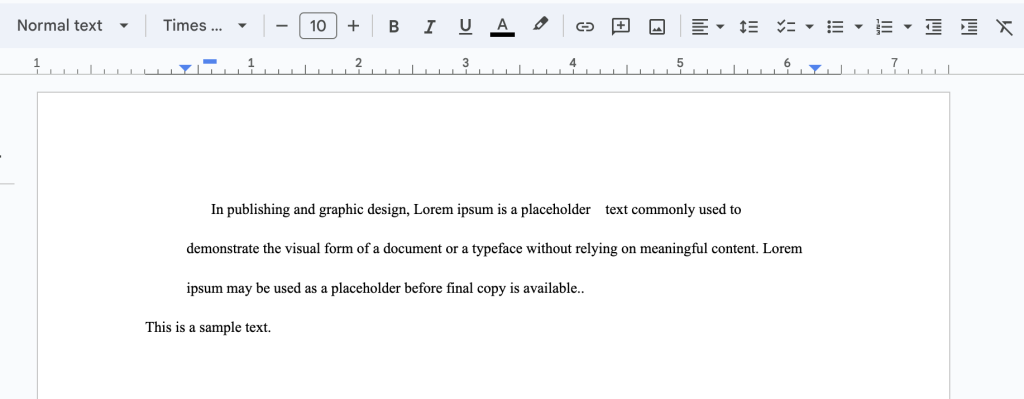
Word Documentでフォントを変更する方法 - 高度な機能
FileFormat.Wordsは、単語文書を変更するためのいくつかの高度なオプションも提供しています。 Docxファイルをさらに編集する方法を見てみましょう。 次の手順とコードスニペットに従うことができます。
- BoldプロパティをTrueに設定して、テキストを太字にする。
- イタリックプロパティの価値を設定することにより、テキストを斜体にします。
- fontfamilyプロパティの値を設定して、テキストのフォントファミリを設定します。
- fontsizeプロパティにアクセスして、フォントサイズを設定します。
- アンダーラインプロパティをTrueに設定して、テキストを強調します。
- 色プロパティは、テキストの色を設定します。
using FileFormat.Words;
namespace Example
{
class Program
{
static void Main(string[] args)
{
// Initialize an instance of the Document class and load an existing Word document.
using (Document doc = new Document("/Users/Mustafa/Desktop/Docs.docx"))
{
//Instantiate the constructor of the Body class with the Document class object.
Body body = new Body(doc);
// Create an object of the Paragraph class.
Paragraph para = new Paragraph();
// Instantiate an instance of the Run class that represents a run of characters in a Word document.
Run run = new Run();
// Access the Text property of the Run class to set the text.
run.Text = "This is a sample text.";
// Set the Bold property to true.
run.Bold = true;
// Make the Text Italic.
run.Italic = true;
// Set the value of FontFamily of the Text.
run.FontFamily = "Algerian";
// Access the FontSize property to set the font size.
run.FontSize = 40;
// Set the Underline property to true to underline the text.
run.Underline = true;
// The Color property will set the color of the text.
run.Color = "FF0000";
// Call the AppendChild() method to attach the object of the Run class with the object of the Paragraph class.
para.AppendChild(run);
// Invoke AppendChild method of the body class to add paragraph to the document.
body.AppendChild(para);
// The Save method will save the Word document onto the disk.
doc.Save("/Docs.docx");
}
}
}
}
メインファイルは、上記のコードスニペットのように見える必要があります。プロジェクトを実行してください。次の出力が表示されます。
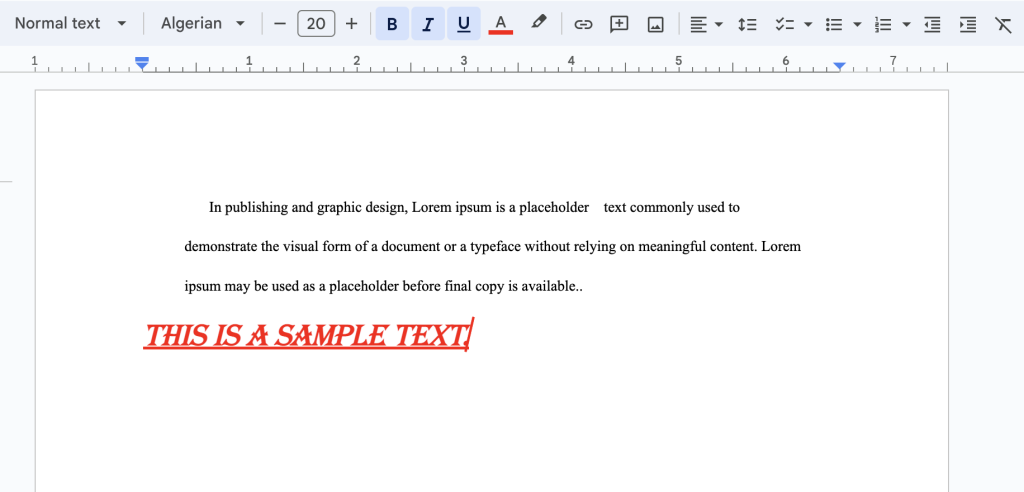
結論
このブログ投稿では、オープンソース.NETライブラリを使用して、C# でWord Documentを編集する方法を学びました。 .netのfileformat.wordsは、プログラムで単語ドキュメントを作成および操作する機能を提供する使いやすいAPIです。さらに、 Word Document でフォントを変更する方法と他のプロパティを変更する方法も実行されました。最後に、この オープンソースDocxエディターの開発と使用に関する包括的なドキュメントがあります。** 最後に、fileformat.comは、他のトピックに関するブログ投稿を書き続けています。だから、最新情報のために連絡を取り合いましょう。さらに、Facebook、LinkedIn、Twitterなど、ソーシャルメディアプラットフォームでフォローできます。
貢献
.netのfileformat.wordsはオープンソースプロジェクトであり、githubで入手可能です。したがって、コミュニティからの貢献は大歓迎です。
質問する
フォーラムでの質問や質問についてお知らせください。
FAQS
** C#を使用してWordドキュメントで書く方法?** .NETプロジェクトにfileformat.words for .netをインストールして、プログラムでdocxファイルを編集することができます。 ** Word Documentを完全に編集するにはどうすればよいですか?** このリンクに従って、C#ライブラリを使用して単語ドキュメントを編集する方法を学習してください。