In our previous article, we introduced the Apache POI components for working with PowerPoint presentation files. We had a look at the HSLF (Horrible Slide Layout Format) and XSLF (XML Slide Layout Format) APIs offered by Apache POI. In this article, we’ll see how to create presentations in Java and save these as PPTX files.
System Requirements
Before you begin, make sure that your system meets the following requirements.
- JDK – Java SE 2 JDK 1.5 or above
- Memory – 1 GB Ram
- Operating System – Windows/ Linux/ Mac OS
Setting Up Development Environment for Apache POI
You’ll need a Java development environment such as Eclipse, IntelliJ IDEA, or any other IDE you’re comfortable with to work with the Apache POI library in your application. Next is to add Apache POI Maven dependency in your application’s pom.xml file as shown below.
Creating Empty Presentation in Java
Now that your development is ready, let’s dive into writing the code for creating our first blank PowerPoint Presentation.
XMLSlideShow pptx = new XMLSlideShow();
File file = new File("fileformat.pptx");
FileOutputStream out = new FileOutputStream(file);
pptx.write(out);
out.close();
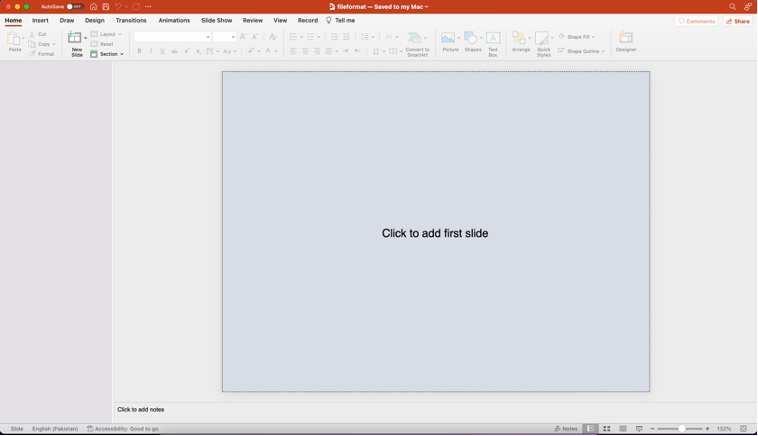
When you open the saved file, you will see that the Presentation opens with Microsoft PowerPoint and doesn’t have any slides in it. This is the default behavior when creating a PowerPoint presentation with Apache POI.
Add Slides to PowerPoint Presentation in Java
The above code sample created an empty PowerPoint presentation without any slides. In order to create a new presentation with slides, use the createSlide method of the XMLSlideShow class as shown in the updated code sample below.
//opening an existing slide show
File file = new File("fileformat.pptx");
FileInputStream inputstream = new FileInputStream(file);
XMLSlideShow ppt = new XMLSlideShow(inputstream);
//adding slides to the slideshow
XSLFSlide slide1 = ppt.createSlide();
XSLFSlide slide2 = ppt.createSlide();
//saving the changes
FileOutputStream out = new FileOutputStream(file);
ppt.write(out);
out.close();
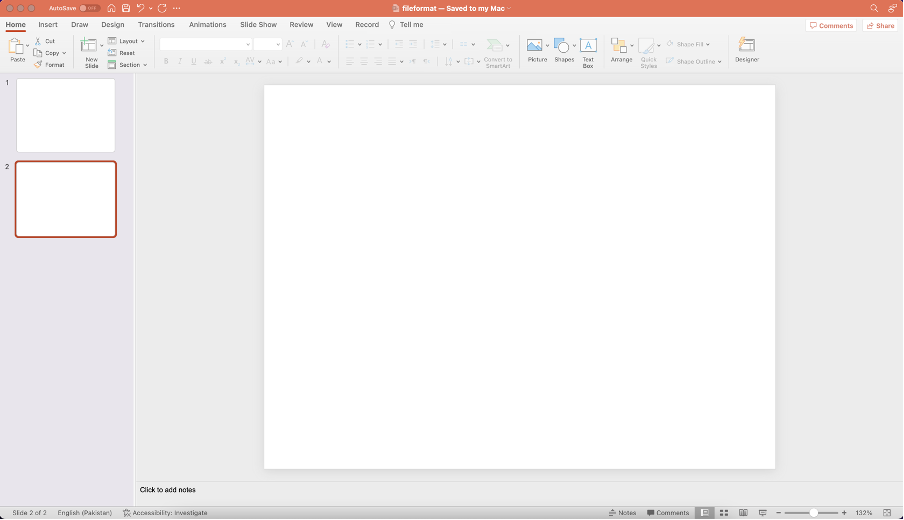
The createSlide method of the XMLSlideShow class is used to add a blank slide to the presentation. This method returns an object of the XSLFSlide class that can be further used to add content to the slide and work with its properties.
Conclusion
Apache POI components for working with PowerPoint presentation files let you create and edit PowerPoint PPT and PPTX files from within your Java application. In our upcoming blogs, we’ll further demonstrate the usage of Apache POI Java components for working with PowerPoint presentations. So, stay tuned.