Headers and Footers are commonly used while working with Word documents. They give a clear view of the main theme of the document as well as any additional information that needs to be put in front of readers. Microsoft Word lets you add header and footer information to your document with customization. If you are a .NET application developer who is interested in developing (or has developed) an application for word document processing, adding the feature of inserting headers and footers to Word documents from your application can be a beneficial feature.
In this article, we’ll go through the steps to add header and footer to a Word document from within a .NET application. We’ll be using NPOI API for .NET in C# for this purpose.
How to Insert Header and Footer to Word Document using Microsoft Word?
Before we can start looking into how to add header and footer information to a Word document from a .NET application, let’s first have a look at how we can do the same using Microsoft Word.
Steps to Insert Header and Footer in Document using Microsoft Word
You can use the following steps to insert header and footer in a DOCX document using Microsoft Word.
- Select Insert > Header or Footer.
- Select one of the built-in designs.
- Type the text you want in the header or footer.
- Select Close Header and Footer when you’re done.
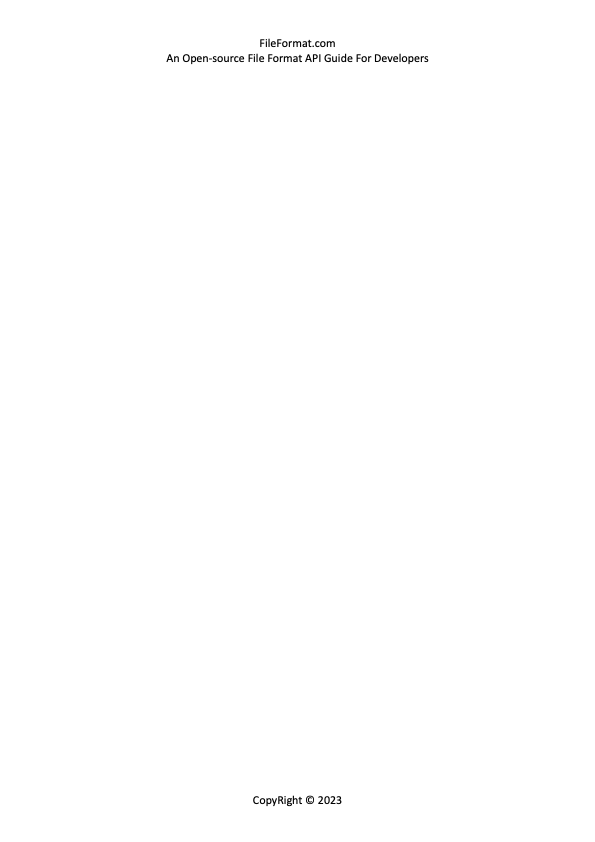
How to Insert Header and Footer using NPOI in C#
Now that we have seen how to insert header and footer in a Word document using Microsoft Word, let’s have a look at how to do the same using NPOI API for .NET in our C# application. Just in case you don’t have much idea about NPOI, you can go through our comprehensive guide for NPOI and its installation guidelines.
Steps to Insert Header and Footer in Word Document using C#
At this stage, we expect that you have created a simple C# console application and added NPOI API to it. Use the following steps to add a header and footer in Word Document using NPOI API in C#.
- Create an instance of XWPFDocument class
- Add instances of XWPFParagraph and XWPFRun to create a paragraph with the required text
- Create a header using CT_Hdr and set its properties and text as shown in the code
- Create a footer using CT_Ftr and set its properties and text as shown in the code
- Create unique relations of the header and footer using XWPFRelation
- Save the file to disc using the XWPFDocument object
//Create document
XWPFDocument doc = new XWPFDocument();
XWPFParagraph para = doc.CreateParagraph();
XWPFRun run = para.CreateRun();
run.SetText("FileFomrat.com");
doc.Document.body.sectPr = new CT_SectPr();
CT_SectPr secPr = doc.Document.body.sectPr;
//Create header and set its text
CT_Hdr header = new CT_Hdr();
//header.AddNewP().AddNewR().AddNewT().Value = "FileFormat.com";
var headerParagraph = header.AddNewP();
var paragraphRun = headerParagraph.AddNewR();
var paragraphText = paragraphRun.AddNewT();
paragraphText.Value = "FileFormat.com - An Open-source File Format API Guide For Developers";
CT_PPr headerPPR = headerParagraph.AddNewPPr();
CT_Jc headerAlign = headerPPR.AddNewJc();
headerAlign.val = ST_Jc.center;
//Create footer and set its text
CT_Ftr footer = new CT_Ftr();
CT_P footerParagraph = footer.AddNewP();
CT_R ctr = footerParagraph.AddNewR();
CT_Text ctt = ctr.AddNewT();
ctt.Value = "CopyRight (C) 2023";
CT_PPr ppr = footerParagraph.AddNewPPr();
CT_Jc align = ppr.AddNewJc();
align.val = ST_Jc.center;
//Create the relation of header
XWPFRelation relation1 = XWPFRelation.HEADER;
XWPFHeader myHeader = (XWPFHeader)doc.CreateRelationship(relation1, XWPFFactory.GetInstance(), doc.HeaderList.Count + 1);
//Create the relation of footer
XWPFRelation relation2 = XWPFRelation.FOOTER;
XWPFFooter myFooter = (XWPFFooter)doc.CreateRelationship(relation2, XWPFFactory.GetInstance(), doc.FooterList.Count + 1);
//Set the header
myHeader.SetHeaderFooter(header);
CT_HdrFtrRef myHeaderRef = secPr.AddNewHeaderReference();
myHeaderRef.type = ST_HdrFtr.@default;
myHeaderRef.id = myHeader.GetXWPFDocument().GetRelationId(myHeader); // = myHeader.GetPackageRelationship().Id;
//Set the footer
myFooter.SetHeaderFooter(footer);
CT_HdrFtrRef myFooterRef = secPr.AddNewFooterReference();
myFooterRef.type = ST_HdrFtr.@default;
myFooterRef.id = myFooter.GetXWPFDocument().GetRelationId(myFooter);//myFooter.GetPackageRelationship().Id;
//Save the file
using (FileStream stream = File.Create("HeaderAndFooter.docx"))
{
doc.Write(stream);
}
Conclusion
In this article, we saw how to add a header and footer to a Word document using NPOI API in C#. We’ll be adding more examples like these to our future articles for working with NPOI API in C# for document processing. So stay tuned.