关注此博客文章以了解如何以编程方式在Word文档中添加表标头。 fileformat.words提供了丰富的表创建和操纵方法。
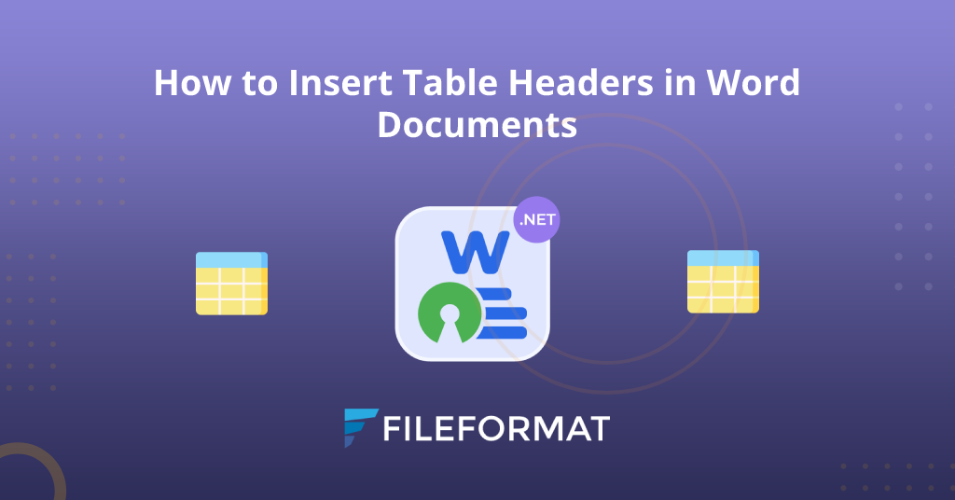
概述
数据表是MS Word文档中的关键元素。使用表是一项例行任务,但是如果有许多文档涉及多个数据表,该怎么办?当然,需要某种自动化来自动化重复任务以节省时间并提高生产力。因此,fileformat.words是一个开源.NET库,可自动化单词创建,修改和处理。在本文中,我们将探讨如何使用此C#API在Word文档中插入表标头。但是,您可以在MS Word 中与 表相关的各种主题访问我们以前的文章。 我们将在此博客文章中浏览以下各节:
使用表标头 - API安装
.NET库的fileformat.words的安装过程是几秒钟的问题。该企业级别.NET API提供了一大堆用户可以利用的功能。因此,您可以下载Nuget软件包或在Nuget软件包管理器中运行以下命令。
Install-Package FileFormat.Words
以编程方式在Word文件中添加表标头
安装完成,下一步是立即编写代码段。此外,我们不仅可以在Word文档中创建表,而且可以通过编程方式自定义表的布局。 您可以按照以下提到的步骤和代码段:
- 实例化文档类的对象。
- 用文档类对象初始化Body类的构造函数。
- 创建表类的实例。
- 通过调用tableheaders方法来设置第一列的标题。
- 调用Append方法将行添加到表中。
- 调用appendchild方法将表添加到文档正文中。
- 保存方法将将Word文档保存到磁盘上。
using FileFormat.Words;
using FileFormat.Words.Table;
namespace Example
{
class Program
{
static void Main(string[] args)
{
// Instantiate an object of the Document class.
using (Document doc = new Document())
{
// Initialize the constructor of the Body class with the Document class object.
Body body = new Body(doc);
// Create an instance of the Table class.
Table table = new Table();
// Initialize the constructor of the TopBorder class to set the border of the top side of the table.
TopBorder topBorder = new TopBorder();
// Invoke the dashed_border method to set the border style and border line width.
topBorder.dashed_border(20);
// To set the border of the bottom side of the table.
BottomBorder bottomBorder = new BottomBorder();
bottomBorder.dashed_border(20);
// To set the border of the right side of the table.
RightBorder rightBorder = new RightBorder();
rightBorder.dashed_border(20);
// To set the border of the left side of the table.
LeftBorder leftBorder = new LeftBorder();
leftBorder.dashed_border(20);
// To set the inside vertical border of the table.
InsideVerticalBorder insideVerticalBorder = new InsideVerticalBorder();
insideVerticalBorder.dashed_border(20);
// To set the inside vehorizontalrtical border of the table.
InsideHorizontalBorder insideHorizontalBorder = new InsideHorizontalBorder();
insideHorizontalBorder.dashed_border(20);
// Create an instance of the TableBorders class.
TableBorders tableBorders = new TableBorders();
// Append the object of the TopBorder class to the object of the TableBorders class.
tableBorders.AppendTopBorder(topBorder);
// Append the object of the BottomBorder class.
tableBorders.AppendBottomBorder(bottomBorder);
// Append the object of the RightBorder class.
tableBorders.AppendRightBorder(rightBorder);
// Append the object of the LeftBorder class.
tableBorders.AppendLeftBorder(leftBorder);
// Append the object of the InsideVerticalBorder class.
tableBorders.AppendInsideVerticalBorder(insideVerticalBorder);
// Append the object of the InsideHorizontalBorder class.
tableBorders.AppendInsideHorizontalBorder(insideHorizontalBorder);
// Initialize an instance of the TableProperties class.
TableProperties tblProp = new TableProperties();
// Invoke the Append method to attach the object of the TableBorders class.
tblProp.Append(tableBorders);
// Create an instance of the TableJustification class
TableJustification tableJustification = new TableJustification();
// Call the AlignLeft method to position the table on left side of the document.
tableJustification.AlignLeft();
// Invoke the Append method to attach the tableJustification object to the tblProp object.
tblProp.Append(tableJustification);
// The AppendChild method will attach the table propertiese to the table.
table.AppendChild(tblProp);
// Create an object of the TableRow class to create a table row.
TableRow tableRow = new TableRow();
TableRow tableRow2 = new TableRow();
// Initialize an istance of the TableCell class.
TableCell tableCell = new TableCell();
Paragraph para = new Paragraph();
Run run = new Run();
// Set the header of the first column by invoking the TableHeaders method.
table.TableHeaders("Country");
run.Text = "England";
para.AppendChild(run);
// Call the Append method to add text inside the table cell.
tableCell.Append(para);
// Create an object of the TableCellProperties table properties
TableCellProperties tblCellProps = new TableCellProperties();
// Set the width of table cell by initializing the object of the TableCellWidth class and append to tblCellProps object.
tblCellProps.Append(new TableCellWidth("2400"));
// Append method will attach the tblCellProps object with the object of the TableCell class.
tableCell.Append(tblCellProps);
TableCell tableCell2 = new TableCell();
Paragraph para2 = new Paragraph();
Run run2 = new Run();
// Invoke the TableHeaders method to set the header of the second column
table.TableHeaders("Capital");
run2.Text = "London";
para2.AppendChild(run2);
tableCell2.Append(para2);
TableCellProperties tblCellProps2 = new TableCellProperties();
tblCellProps2.Append(new TableCellWidth("1400"));
tableCell2.Append(tblCellProps2);
TableCell tableCell3 = new TableCell();
Paragraph para3 = new Paragraph();
Run run3 = new Run();
table.TableHeaders("Population");
run3.Text = "1000000";
para3.AppendChild(run3);
tableCell3.Append(para3);
TableCellProperties tblCellProps3 = new TableCellProperties();
tblCellProps3.Append(new TableCellWidth("1400"));
tableCell3.Append(tblCellProps3);
// Call the Append method to add cells into table row.
tableRow.Append(tableCell);
tableRow.Append(tableCell2);
tableRow.Append(tableCell3);
// create table cell
TableCell _tableCell = new TableCell();
Paragraph _para = new Paragraph();
Run _run = new Run();
_run.Text = "Pakistan";
_para.AppendChild(_run);
_tableCell.Append(_para);
TableCellProperties tblCellProps1_ = new TableCellProperties();
tblCellProps1_.Append(new TableCellWidth("2400"));
_tableCell.Append(tblCellProps1_);
TableCell _tableCell2 = new TableCell();
Paragraph _para2 = new Paragraph();
Run _run2 = new Run();
_run2.Text = "Islamabad";
_para2.AppendChild(_run2);
_tableCell2.Append(_para2);
TableCellProperties tblCellProps2_ = new TableCellProperties();
tblCellProps2_.Append(new TableCellWidth("1400"));
_tableCell2.Append(tblCellProps2_);
TableCell _tableCell3 = new TableCell();
Paragraph _para3 = new Paragraph();
Run _run3 = new Run();
_run3.Text = "2000000";
_para3.AppendChild(_run3);
_tableCell3.Append(_para3);
TableCellProperties tblCellProps3_ = new TableCellProperties();
tblCellProps3_.Append(new TableCellWidth("1400"));
_tableCell3.Append(tblCellProps3_);
tableRow2.Append(_tableCell);
tableRow2.Append(_tableCell2);
tableRow2.Append(_tableCell3);
// Invoke the Append method to add the rows into table.
table.Append(tableRow);
table.Append(tableRow2);
// Call the AppendChild method to add the table to the body of the document.
body.AppendChild(table);
// The Save method will save the Word document onto the disk.
doc.Save("/Users/Mustafa/Desktop/Docs.docx");
}
}
}
}
将上述代码复制并粘贴到主文件中并运行程序。您将看到下图中显示的输出:
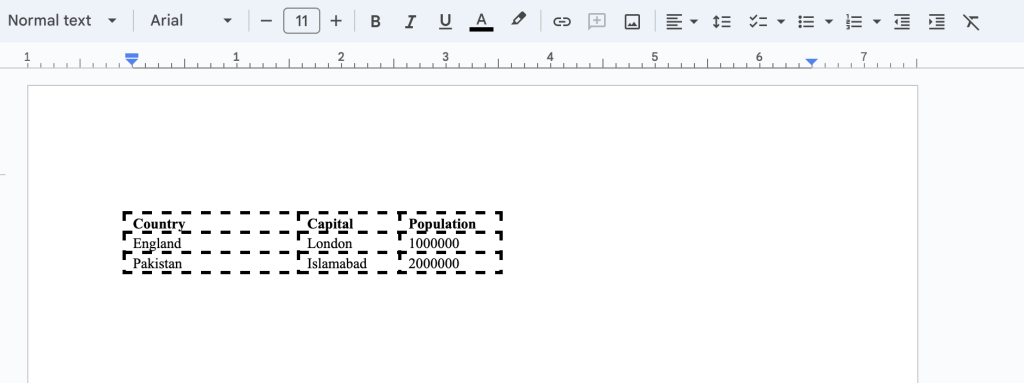
结论
我们正在此处结束这篇博客文章,希望您学习如何使用fileformat.words库在Word文档中插入表标头。此外,您还完成了安装过程和代码段。此外,您可以在文档中探索其他实用方法。 最后,fileformat.com继续写有关其他主题的博客文章。此外,您可以在我们的社交媒体平台上关注我们,包括Facebook,LinkedIn和Twitter。
贡献
由于.NET的FileFormat.Words是一个开源项目,可在GitHub上找到。因此,社区的贡献非常感谢。
问一个问题
您可以在我们的论坛上让我们知道您的问题或查询。
常见问题 - 常见问题
如何用标头插入桌子? 请按照此链接学习如何在C#中插入表标头。